API Reference
This section is a reference for the Open GoPro Python Package API. The BLE / Wifi API’s that this package implements can be found in the Open GoPro documentation linked from package summary
Note
Not everything is exposed here. This section should only consist of the interface information that a user (not a developer) of the Open GoPro module should care about.
For a higher-level summary / usage, see the usage section
Warning
This documentation is not a substitute for the Open GoPro BLE and WiFi specifications. That is, this interface shows how to use the various commands but is not an exhaustive source of information for what each command does. The Open GoPro specs should be used simultaneously with this document for development.
GoPro Client
There are two top-level GoPro client interfaces - Wireless and Wired:
Wireless
from open_gopro import WirelessGoPro
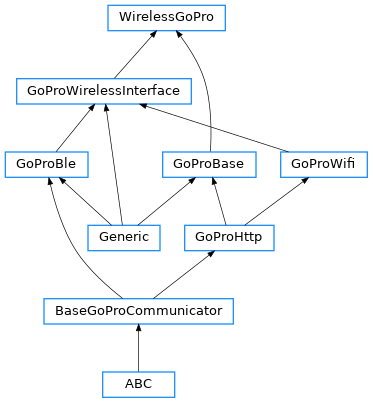
- class WirelessGoPro(target: Pattern | None = None, wifi_interface: str | None = None, sudo_password: str | None = None, enable_wifi: bool = True, **kwargs: Any)
The top-level BLE and Wifi interface to a Wireless GoPro device.
See the Open GoPro SDK for complete documentation.
This will handle, for BLE:
discovering target GoPro device
establishing the connection
discovering GATT characteristics
enabling notifications
discovering Open GoPro version
setting the date, time, timezone, and DST
transferring data
This will handle, for Wifi:
finding SSID and password
establishing Wifi connection
transferring data
It will also do some synchronization, etc:
ensuring camera is ready / not encoding before transferring data
sending keep alive signal periodically
If no target arg is passed in, the first discovered BLE GoPro device will be connected to.
It can be used via context manager:
>>> async with WirelessGoPro() as gopro: >>> print("Yay! I'm connected via BLE, Wifi, opened, and ready to send / get data now!") >>> # Send some messages now
Or without:
>>> gopro = WirelessGoPro() >>> await gopro.open() >>> print("Yay! I'm connected via BLE, Wifi, opened, and ready to send / get data now!") >>> # Send some messages now
- Parameters:
target (Pattern | None) – A regex to search for the target GoPro’s name. For example, “GoPro 0456”). Defaults to None (i.e. connect to first discovered GoPro)
wifi_interface (str | None) – Set to specify the wifi interface the local machine will use to connect to the GoPro. If None (or not set), first discovered interface will be used.
sudo_password (str | None) – User password for sudo. If not passed, you will be prompted if a password is needed which should only happen on Nix systems.
enable_wifi (bool) – Optionally do not enable Wifi if set to False. Defaults to True.
**kwargs (Any) – additional parameters for internal use / testing
# noqa: DAR401
- Raises:
InterfaceConfigFailure – In order to communicate via Wifi, there must be an available # noqa: DAR402 Wifi Interface. By default during initialization, the Wifi driver will attempt to automatically discover such an interface. If it does not find any, it will raise this exception. Note that the interface can also be specified manually with the ‘wifi_interface’ argument.
- property ble_command: BleCommands
Used to call the BLE commands
- Returns:
the commands
- Return type:
- property ble_setting: BleSettings
Used to access the BLE settings
- Returns:
the settings
- Return type:
- property ble_status: BleStatuses
Used to access the BLE statuses
- Returns:
the statuses
- Return type:
- async close() None
Safely stop the GoPro instance.
This will disconnect BLE and WiFI if applicable.
If not using the context manager, it is mandatory to call this before exiting the program in order to prevent reconnection issues because the OS has never disconnected from the previous session.
- async configure_cohn(timeout: int = 60) bool
Prepare Camera on the Home Network
Provision if not provisioned Then wait for COHN to be connected and ready
- async connect_to_access_point(ssid: str, password: str) bool
Connect the camera to a Wifi Access Point
- property http_command: HttpCommands
Used to access the Wifi commands
- Returns:
the commands
- Return type:
- property http_setting: HttpSettings
Used to access the Wifi settings
- Returns:
the settings
- Return type:
- property identifier: str
Get a unique identifier for this instance.
The identifier is the last 4 digits of the camera. That is, the same string that is used to scan for the camera for BLE.
If no target has been provided and a camera is not yet found, this will be None
- Raises:
GoProNotOpened – Client is not opened yet so no identifier is available
- Returns:
last 4 digits if available, else None
- Return type:
- property is_ble_connected: bool
Are we connected via BLE to the GoPro device?
- Returns:
True if yes, False if no
- Return type:
- property is_cohn_provisioned: bool
Is COHN currently provisioned?
Get the current COHN status from the camera
- Returns:
True if COHN is provisioned, False otherwise
- Return type:
- property is_http_connected: bool
Are we connected via HTTP to the GoPro device?
- Returns:
True if yes, False if no
- Return type:
- property is_open: bool
Is this client ready for communication?
- Returns:
True if yes, False if no
- Return type:
- property is_ready: bool
Is gopro ready to receive commands
- Returns:
yes if ready, no otherwise
- Return type:
- async keep_alive() bool
Send a heartbeat to prevent the BLE connection from dropping.
This is sent automatically by the GoPro instance if its maintain_ble argument is not False.
- Returns:
True if it succeeded,. False otherwise
- Return type:
- async open(timeout: int = 15, retries: int = 5) None
Perform all initialization commands for ble and wifi
For BLE: scan and find device, establish connection, discover characteristics, configure queries start maintenance, and get Open GoPro version..
For Wifi: discover SSID and password, enable and connect. Or disable if not using.
- Raises:
Exception – Any exceptions during opening are propagated through
InvalidOpenGoProVersion – Only 2.0 is supported
- Parameters:
- property password: str | None
Get the GoPro AP’s password
- Returns:
password or None if it is not known
- Return type:
str | None
- register_update(callback: Callable[[SettingId | StatusId | ActionId, Any], Coroutine[Any, Any, None]], update: SettingId | StatusId | ActionId) None
Register for callbacks when an update occurs
- Parameters:
callback (UpdateCb) – callback to be notified in
update (UpdateType) – update to register for
- property ssid: str | None
Get the GoPro AP’s WiFi SSID
- Returns:
SSID or None if it is not known
- Return type:
str | None
- unregister_update(callback: Callable[[SettingId | StatusId | ActionId, Any], Coroutine[Any, Any, None]], update: SettingId | StatusId | ActionId | None = None) None
Unregister for asynchronous update(s)
- Parameters:
callback (UpdateCb) – callback to stop receiving update(s) on
update (UpdateType | None) – updates to unsubscribe for. Defaults to None (all updates that use this callback will be unsubscribed).
Wired
from open_gopro import WiredGoPro
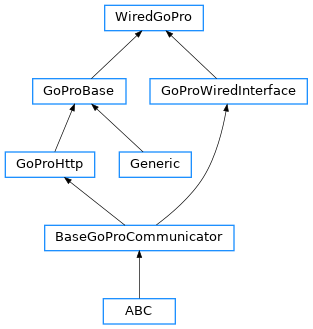
- class WiredGoPro(serial: str | None = None, **kwargs: Any)
The top-level USB interface to a Wired GoPro device.
See the Open GoPro SDK for complete documentation.
If a serial number is not passed when instantiating, the mDNS server will be queried to find a connected GoPro.
- This class also handles:
ensuring camera is ready / not encoding before transferring data
It can be used via context manager:
>>> async with WiredGoPro() as gopro: >>> print("Yay! I'm connected via USB, opened, and ready to send / get data now!") >>> # Send some messages now
Or without:
>>> gopro = WiredGoPro() >>> await gopro.open() >>> print("Yay! I'm connected via USB, opened, and ready to send / get data now!") >>> # Send some messages now
- Parameters:
serial (str | None) – (at least) last 3 digits of GoPro Serial number. If not set, first GoPro discovered from mDNS will be used. Defaults to None
**kwargs (Any) – additional keyword arguments to pass to base class
- property ble_command: BleCommands
Used to call the BLE commands
- Raises:
NotImplementedError – Not valid for WiredGoPro
- property ble_setting: BleSettings
Used to access the BLE settings
- Raises:
NotImplementedError – Not valid for WiredGoPro
- property ble_status: BleStatuses
Used to access the BLE statuses
- Raises:
NotImplementedError – Not valid for WiredGoPro
- async configure_cohn(timeout: int = 60) bool
Prepare Camera on the Home Network
Provision if not provisioned Then wait for COHN to be connected and ready
- Parameters:
timeout (int) – time in seconds to wait for COHN to be ready. Defaults to 60.
- Returns:
True if success, False otherwise
- Return type:
- Raises:
NotImplementedError – not yet possible
- property http_command: HttpCommands
Used to access the USB commands
- Returns:
the commands
- Return type:
- property http_setting: HttpSettings
Used to access the USB settings
- Returns:
the settings
- Return type:
- property identifier: str
Unique identifier for the connected GoPro Client
- Raises:
GoProNotOpened – serial was not passed to instantiation and IP has not yet been discovered
- Returns:
identifier
- Return type:
- property is_ble_connected: bool
Are we connected via BLE to the GoPro device?
- Returns:
True if yes, False if no
- Return type:
- property is_cohn_provisioned: bool
Is COHN currently provisioned?
Get the current COHN status from the camera
- Returns:
True if COHN is provisioned, False otherwise
- Return type:
- Raises:
NotImplementedError – not yet possible
- property is_http_connected: bool
Are we connected via Wifi to the GoPro device?
- Returns:
True if yes, False if no
- Return type:
- property is_open: bool
Is this client ready for communication?
- Returns:
True if yes, False if no
- Return type:
- property is_ready: bool
Is gopro ready to receive commands
- Returns:
yes if ready, no otherwise
- Return type:
- async open(timeout: int = 10, retries: int = 1) None
Connect to the Wired GoPro Client and prepare it for communication
- Parameters:
# noqa: DAR401
- Raises:
InvalidOpenGoProVersion – the GoPro camera does not support the correct Open GoPro API version
FailedToFindDevice – could not auto-discover GoPro via mDNS # noqa: DAR402
- register_update(callback: Callable[[SettingId | StatusId | ActionId, Any], Coroutine[Any, Any, None]], update: SettingId | StatusId | ActionId) None
Register for callbacks when an update occurs
- Parameters:
callback (UpdateCb) – callback to be notified in
update (UpdateType) – update to register for
- Raises:
NotImplementedError – not yet possible
- unregister_update(callback: Callable[[SettingId | StatusId | ActionId, Any], Coroutine[Any, Any, None]], update: SettingId | StatusId | ActionId | None = None) None
Unregister for asynchronous update(s)
- Parameters:
callback (UpdateCb) – callback to stop receiving update(s) on
update (UpdateType | None) – updates to unsubscribe for. Defaults to None (all updates that use this callback will be unsubscribed).
- Raises:
NotImplementedError – not yet possible
Open GoPro API
These are both the base types that are used to implement the API (BLE Setting, Ble Status, etc.) and the version-specific API’s themselves.
These should not be imported directly and instead should be accessed using the relevant properties (ble_command,
wifi_setting, etc.) of a GoPro(open_gopro.gopro_base.GoProBase
) instance.
- class BleCommands(communicator: CommunicatorType)
Bases:
BleMessages
[BleMessage
]All of the BLE commands.
To be used as a delegate for a GoProBle instance to build commands
- async cohn_clear_certificate() GoProResp[None]
Clear the current SSL certificate on the camera that is used for COHN
- Returns:
was the clear successful?
- Return type:
GoProResp[None]
- async cohn_create_certificate(*, override: bool = False) GoProResp[None]
Create an SSL certificate on the camera to use for COHN
- async cohn_get_certificate() GoProResp[ResponseCOHNCert]
Get the current SSL certificate that the camera is using for COHN.
- Returns:
the certificate
- Return type:
GoProResp[proto.ResponseCOHNCert]
- async cohn_get_status(*, register: bool) GoProResp[NotifyCOHNStatus]
Get (and optionally register for) the current COHN status
- async cohn_set_setting(*, mode: Toggle) GoProResp[None]
Set a COHN specific setting.
- Parameters:
mode (Params.Toggle) – should camera auto connect to home network?
- Returns:
status of set
- Return type:
GoProResp[None]
- async custom_preset_update(icon_id: int | None = None, title: str | int | None = None) GoProResp[ResponseGeneric]
Update a custom preset title and / or icon
- Parameters:
icon_id (proto.EnumPresetIcon.ValueType | None) – Icon ID. Defaults to None.
title (str | proto.EnumPresetTitle.ValueType | None) – Custom Preset name or Factory Title ID. Defaults to None.
- Raises:
ValueError – Did not set a parameter
TypeError – Title was not proto.EnumPresetTitle.ValueType or string
- Returns:
status of preset update
- Return type:
GoProResp[proto.ResponseGeneric]
- async get_ap_entries(*, scan_id: int, start_index: int = 0, max_entries: int = 100) GoProResp[ResponseGetApEntries]
Get the results of a scan for wifi networks
- Parameters:
- Returns:
result of scan with entries for WiFi networks
- Return type:
GoProResp[proto.ResponseGetApEntries]
- async get_camera_capabilities() GoProResp[dict[SettingId | StatusId, Any]]
Get the current capabilities of each camera setting
- Returns:
response as JSON
- Return type:
- async get_camera_settings() GoProResp[dict[SettingId | StatusId, Any]]
Get all of the camera’s settings
- Returns:
response as JSON
- Return type:
- async get_camera_statuses() GoProResp[dict[SettingId | StatusId, Any]]
Get all of the camera’s statuses
- Returns:
response as JSON
- Return type:
- async get_date_time() GoProResp[datetime]
Get the camera’s date and time (non timezone / DST version)
- Returns:
response as JSON
- Return type:
- async get_date_time_tz_dst() GoProResp[TzDstDateTime]
Get the camera’s date and time with timezone / DST
- Returns:
response as JSON
- Return type:
- async get_hardware_info() GoProResp[CameraInfo]
Get the model number, board, type, firmware version, serial number, and AP info
- Returns:
response as JSON
- Return type:
- async get_last_captured_media() GoProResp[ResponseLastCapturedMedia]
Get the last captured media file
- Returns:
status of request and last captured file if successful
- Return type:
GoProResp[proto.ResponseLastCapturedMedia]
- async get_preset_status(*, register: list[int] | None = None, unregister: list[int] | None = None) GoProResp[NotifyPresetStatus]
Get information about what Preset Groups and Presets the camera supports in its current state
Also optionally (un)register for preset / group preset modified notifications which will be sent asynchronously as
open_gopro.constants.ActionId.PRESET_MODIFIED_NOTIFICATION
- Parameters:
- Returns:
JSON data describing all currently available presets
- Return type:
GoProResp[proto.NotifyPresetStatus]
- async load_preset(*, preset: int) GoProResp[None]
Load a Preset
The integer preset value can be found from the get_preset_status command
- async load_preset_group(*, group: int) GoProResp[None]
Load a Preset Group.
Once complete, the most recently used preset in this group will be active.
- Parameters:
group (proto.EnumPresetGroup.ValueType) – preset group to load
- Returns:
response as JSON
- Return type:
GoProResp[None]
- async power_down() GoProResp[None]
Power Down the camera
- Returns:
status of command
- Return type:
GoProResp[None]
- async register_for_all_capabilities(callback: Callable[[SettingId | StatusId | ActionId, Any], Coroutine[Any, Any, None]]) GoProResp[None]
Register push notifications for all capabilities
- async register_for_all_settings(callback: Callable[[SettingId | StatusId | ActionId, Any], Coroutine[Any, Any, None]]) GoProResp[None]
Register push notifications for all settings
- async register_for_all_statuses(callback: Callable[[SettingId | StatusId | ActionId, Any], Coroutine[Any, Any, None]]) GoProResp[None]
Register push notifications for all statuses
- async register_livestream_status(*, register: list[int] | None = None, unregister: list[int] | None = None) GoProResp[NotifyLiveStreamStatus]
Register / unregister to receive asynchronous livestream statuses
- Parameters:
- Returns:
current livestream status
- Return type:
GoProResp[proto.NotifyLiveStreamStatus]
- async release_network() GoProResp[None]
Disconnect the camera Wifi network in STA mode so that it returns to AP mode.
- Returns:
status of release request
- Return type:
GoProResp[None]
- async request_wifi_connect(*, ssid: str) GoProResp[ResponseConnect]
Request the camera to connect to a WiFi network that is already provisioned.
Updates will be sent as
open_gopro.constants.ActionId.NOTIF_PROVIS_STATE
- async request_wifi_connect_new(*, ssid: str, password: str) GoProResp[ResponseConnectNew]
Request the camera to connect to a WiFi network that is not already provisioned.
Updates will be sent as
open_gopro.constants.ActionId.NOTIF_PROVIS_STATE
- async scan_wifi_networks() GoProResp[ResponseStartScanning]
Scan for Wifi networks
- Returns:
Command status of request
- Return type:
GoProResp[proto.ResponseStartScanning]
- async set_camera_control(*, camera_control_status: int) GoProResp[None]
Tell the camera that the app (i.e. External Control) wishes to claim control of the camera.
- Parameters:
camera_control_status (proto.EnumCameraControlStatus.ValueType) – Desired camera control.
- Returns:
command status of request
- Return type:
GoProResp[None]
- async set_date_time(*, date_time: datetime) GoProResp[None]
Set the camera’s date and time (non timezone / DST version)
- Parameters:
date_time (datetime.datetime) – Date and time to set (Timezone will be ignored)
- Returns:
command status
- Return type:
GoProResp[None]
- async set_date_time_tz_dst(*, date_time: datetime, tz_offset: int, is_dst: bool) GoProResp[None]
Set the camera’s date and time with timezone and DST
- Parameters:
date_time (datetime.datetime) – date and time
tz_offset (int) – timezone as UTC offset
is_dst (bool) – is daylight savings time?
- Returns:
command status
- Return type:
GoProResp[None]
- async set_livestream_mode(*, url: str, minimum_bitrate: int, maximum_bitrate: int, starting_bitrate: int, window_size: int | None = None, lens: int | None = None, certs: list[Path] | None = None) GoProResp[None]
Initiate livestream to any site that accepts an RTMP URL and simultaneously encode to camera.
- Parameters:
url (str) – url used to stream. Set to empty string to invalidate/cancel stream
minimum_bitrate (int) – Desired minimum streaming bitrate (>= 800)
maximum_bitrate (int) – Desired maximum streaming bitrate (<= 8000)
starting_bitrate (int) – Initial streaming bitrate (honored if 800 <= value <= 8000)
window_size (proto.EnumWindowSize.ValueType | None) – Streaming video resolution. Defaults to None (use camera default).
lens (proto.EnumLens.ValueType | None) – Streaming Field of View. Defaults to None (use camera default).
certs (list[Path] | None) – list of certificates to use. Defaults to None.
- Returns:
command status of request
- Return type:
GoProResp[None]
- async set_shutter(*, shutter: Toggle) GoProResp[None]
Set the Shutter to start / stop encoding
- Parameters:
shutter (Params.Toggle) – on or off
- Returns:
status of command
- Return type:
GoProResp[None]
- async set_third_party_client_info() GoProResp[None]
Flag as third party app
- Returns:
command status
- Return type:
GoProResp[None]
- async set_turbo_mode(*, mode: Toggle) GoProResp[None]
Enable / disable turbo mode.
- Parameters:
mode (Params.Toggle) – True to enable, False to disable.
- Returns:
command status of request
- Return type:
GoProResp[None]
- async sleep() GoProResp[None]
Put the camera in standby
- Returns:
status of command
- Return type:
GoProResp[None]
- async tag_hilight() GoProResp[None]
Tag a highlight during encoding
- Returns:
status of command
- Return type:
GoProResp[None]
- async unregister_for_all_capabilities(callback: Callable[[SettingId | StatusId | ActionId, Any], Coroutine[Any, Any, None]]) GoProResp[None]
Unregister push notifications for all capabilities
- async unregister_for_all_settings(callback: Callable[[SettingId | StatusId | ActionId, Any], Coroutine[Any, Any, None]]) GoProResp[None]
Unregister push notifications for all settings
- class BleSettings(communicator: GoProBle)
Bases:
BleMessages
[BleSettingMessageBase
]The collection of all BLE Settings.
To be used by a GoProBle delegate to build setting messages.
- Parameters:
communicator (GoProBle) – Adapter to read / write settings
- anti_flicker: BleSettingFacade[AntiFlicker]
Anti Flicker frequency.
- auto_off: BleSettingFacade[AutoOff]
Set the auto off time.
- bit_depth: BleSettingFacade[BitDepth]
System Video Bit depth.
- bit_rate: BleSettingFacade[BitRate]
System Video Bit Rate.
- camera_ux_mode: BleSettingFacade[CameraUxMode]
Camera controls configuration.
- fps: BleSettingFacade[FPS]
Frames per second.
- framing: BleSettingFacade[Framing]
Video Framing Mode
- hindsight: BleSettingFacade[Hindsight]
Hindsight time / disable
- hypersmooth: BleSettingFacade[HypersmoothMode]
Set / disable hypersmooth.
- led: BleSettingFacade[LED]
Set the LED options (or also send the BLE keep alive signal).
- max_lens_mode: BleSettingFacade[MaxLensMode]
Enable / disable max lens mod.
- maxlens_mod_type: BleSettingFacade[MaxLensModType]
Max lens mod? If so, what type?
- maxlens_status: BleSettingFacade[Toggle]
Enable / disable max lens mod
- media_format: BleSettingFacade[MediaFormat]
Set the media format.
- multi_shot_easy_aspect_ratio: BleSettingFacade[EasyAspectRatio]
Multi shot easy aspect ratio
- multi_shot_field_of_view: BleSettingFacade[MultishotFOV]
Multi-shot FOV.
- multi_shot_nlv_aspect_ratio: BleSettingFacade[EasyAspectRatio]
Multi shot NLV aspect ratio
- photo_duration: BleSettingFacade[PhotoDuration]
Interval between photo captures
- photo_easy_mode: BleSettingFacade[PhotoEasyMode]
Night Photo easy mode.
- photo_field_of_view: BleSettingFacade[PhotoFOV]
Photo FOV.
- photo_horizon_leveling: BleSettingFacade[HorizonLeveling]
Lock / unlock horizon leveling for photo.
- photo_interval: BleSettingFacade[PhotoInterval]
Interval between photo captures
- photo_mode: BleSettingFacade[PhotoMode]
Photo Mode
- photo_output: BleSettingFacade[PhotoOutput]
File type of photo output
- resolution: BleSettingFacade[Resolution]
Resolution.
- star_trail_length: BleSettingFacade[StarTrailLength]
Multi shot star trail length.
- system_video_mode: BleSettingFacade[SystemVideoMode]
System video mode.
- timelapse_mode: BleSettingFacade[TimelapseMode]
Timelapse Mode
- video_aspect_ratio: BleSettingFacade[VideoAspectRatio]
Video aspect ratio
- video_duration: BleSettingFacade[VideoDuration]
If set, a video will automatically be stopped after recording for this long.
- video_easy_aspect_ratio: BleSettingFacade[EasyAspectRatio]
Video easy aspect ratio
- video_easy_mode: BleSettingFacade[int]
Video easy mode speed. It is not feasible to maintain this setting without code generation so just read as int.
- video_field_of_view: BleSettingFacade[VideoFOV]
Video FOV.
- video_horizon_leveling: BleSettingFacade[HorizonLeveling]
Lock / unlock horizon leveling for video.
- video_mode: BleSettingFacade[VideoMode]
Video Mode (i.e. quality)
- video_performance_mode: BleSettingFacade[PerformanceMode]
Video Performance Mode.
- video_profile: BleSettingFacade[VideoProfile]
Video Profile (hdr, etc.)
- wifi_band: BleSettingFacade[WifiBand]
Current WiFi band being used.
- class BleStatuses(communicator: GoProBle)
Bases:
BleMessages
[BleStatusMessageBase
]All of the BLE Statuses.
To be used by a GoProBle delegate to build status messages.
- Parameters:
communicator (GoProBle) – Adapter to read / write settings
- acc_mic_stat: BleStatusFacade[ExposureMode]
Microphone Accessory status.
- active_preset: BleStatusFacade[int]
Currently Preset (ID).
- analytics_rdy: BleStatusFacade[AnalyticsState]
The current state of camera analytics.
- analytics_size: BleStatusFacade[int]
The size (units??) of the analytics file.
- ap_ssid: BleStatusFacade[str]
Camera’s WIFI SSID. On BLE connection, value is big-endian byte-encoded int.
- ap_state: BleStatusFacade[bool]
Is the Wifi radio enabled?
- app_count: BleStatusFacade[int]
The number of wireless devices connected to the camera.
- band_5ghz_avail: BleStatusFacade[bool]
Is 5GHz wireless band available?
- batt_level: BleStatusFacade[int]
Rough approximation of internal battery level in bars.
- batt_ok_ota: BleStatusFacade[bool]
Is the internal battery charged sufficiently to start Over The Air (OTA) update?
- batt_present: BleStatusFacade[bool]
Is the system’s internal battery present?
- camera_control: BleStatusFacade[CameraControl]
Camera control status ID
- camera_lens_mod: BleStatusFacade[LensModStatus]
Current camera lens mod
- camera_lens_type: BleStatusFacade[MaxLensMode]
Camera lens type (reflects changes to setting 162).
- capt_delay_active: BleStatusFacade[bool]
Is Capture Delay currently active (i.e. counting down)?
- control_allowed_over_usb: BleStatusFacade[bool]
Is control allowed over USB?
- creating_preset: BleStatusFacade[bool]
Is the camera in the process of creating a custom preset?
- current_time_ms: BleStatusFacade[int]
System time in milliseconds since system was booted.
- dig_zoom_active: BleStatusFacade[bool]
Is Digital Zoom feature available?
- digital_zoom: BleStatusFacade[int]
Digital Zoom level (percent).
- download_cancel_pend: BleStatusFacade[bool]
Is download firmware update cancel request pending?
- encoding_active: BleStatusFacade[bool]
Is the camera currently encoding (i.e. capturing photo / video)?
- exposure_type: BleStatusFacade[ExposureMode]
Liveview Exposure Select Mode.
- exposure_x: BleStatusFacade[int]
Liveview Exposure Select for y-coordinate (percent).
- exposure_y: BleStatusFacade[int]
Liveview Exposure Select for y-coordinate (percent).
- first_time: BleStatusFacade[bool]
Is the camera currently in First Time Use (FTU) UI flow?
- flatmode_id: BleStatusFacade[Flatmode]
Current flatmode ID.
- gps_stat: BleStatusFacade[bool]
Does the camera currently have a GPS lock?
Is the camera currently in a contextual menu (e.g. Preferences)?
- int_batt_per: BleStatusFacade[int]
Internal battery level (percent).
- last_hilight: BleStatusFacade[int]
Time since boot (msec) of most recent hilight in encoding video (set to 0 when encoding stops).
- lcd_lock_active: BleStatusFacade[bool]
Is the LCD lock currently active?
- linux_core_active: BleStatusFacade[bool]
Is the system’s Linux core active?
- live_burst_rem: BleStatusFacade[int]
How many Live Bursts can be captured before sdcard is full?
- live_burst_total: BleStatusFacade[int]
Total number of Live Bursts on sdcard.
- locate_active: BleStatusFacade[bool]
Is locate camera feature active?
- logs_ready: BleStatusFacade[bool]
Are system logs ready to be downloaded?
- media_mod_mic_stat: BleStatusFacade[MediaModMicStatus]
Media mod State.
- media_mod_stat: BleStatusFacade[MediaModStatus]
Media Mode Status (bitmasked).
- mobile_video: BleStatusFacade[bool]
Are current video settings mobile friendly? (related to video compression and frame rate).
- mode_group: BleStatusFacade[int]
Current mode group (deprecated in HERO8).
- multi_count_down: BleStatusFacade[int]
The current timelapse interval countdown value (e.g. 5…4…3…2…1…).
- next_poll: BleStatusFacade[int]
The min time between camera status updates (msec). Do not poll for status more often than this.
- num_group_photo: BleStatusFacade[int]
How many group photos can be taken with current settings before sdcard is full?
- num_group_video: BleStatusFacade[int]
Total number of group videos on sdcard.
- num_hilights: BleStatusFacade[int]
The number of hilights in encoding video (set to 0 when encoding stops).
- num_total_photo: BleStatusFacade[int]
Total number of photos on sdcard.
- num_total_video: BleStatusFacade[int]
Total number of videos on sdcard.
- orientation: BleStatusFacade[Orientation]
The rotational orientation of the camera.
- ota_stat: BleStatusFacade[OTAStatus]
The current status of Over The Air (OTA) update.
- pair_state: BleStatusFacade[PairState]
What is the pair state?
- pair_state2: BleStatusFacade[int]
Wireless Pairing State.
- pair_time: BleStatusFacade[int]
Time (milliseconds) since boot of last successful pairing complete action.
- pair_type: BleStatusFacade[PairType]
The last type of pairing that the camera was engaged in.
- photo_interval_capture_count: BleStatusFacade[int]
Photo interval capture count
- photo_presets: BleStatusFacade[int]
Current Photo Preset (ID).
- photos_rem: BleStatusFacade[int]
How many photos can be taken before sdcard is full?
- preset_modified: BleStatusFacade[int]
Preset Modified Status, which contains an event ID and a preset (group) ID.
- presets_group: BleStatusFacade[int]
Current Preset Group (ID).
- preview_enabled: BleStatusFacade[bool]
Is preview stream enabled?
- quick_capture: BleStatusFacade[bool]
Is quick capture feature enabled?
- remote_ctrl_conn: BleStatusFacade[bool]
Is the remote control connected?
- remote_ctrl_ver: BleStatusFacade[int]
What is the remote control version?
- scheduled_capture: BleStatusFacade[bool]
Is Scheduled Capture set?
- scheduled_preset: BleStatusFacade[int]
Scheduled Capture Preset ID.
- sd_rating_check_error: BleStatusFacade[bool]
Does sdcard meet specified minimum write speed?
- sd_status: BleStatusFacade[SDStatus]
Primary Storage Status.
- sd_write_speed_error: BleStatusFacade[int]
Number of sdcard write speed errors since device booted
- sec_sd_stat: BleStatusFacade[SDStatus]
Secondary Storage Status (exclusive to Superbank).
- space_rem: BleStatusFacade[int]
Remaining space on the sdcard in Kilobytes.
- streaming_supp: BleStatusFacade[bool]
Is streaming supports in current recording/flatmode/secondary-stream?
- system_busy: BleStatusFacade[bool]
Is the camera busy?
- system_hot: BleStatusFacade[bool]
Is the system currently overheating?
- system_ready: BleStatusFacade[bool]
Is the system ready to accept messages?
- timelapse_presets: BleStatusFacade[int]
Current Timelapse Preset (ID).
- timelapse_rem: BleStatusFacade[int]
How many min of Timelapse video can be captured with current settings before sdcard is full?
- timewarp_speed_ramp: BleStatusFacade[TimeWarpSpeed]
Time Warp Speed.
- total_sd_space_kb: BleStatusFacade[int]
Total space taken up on the SD card in kilobytes
- turbo_mode: BleStatusFacade[bool]
Is Turbo Transfer active?
- usb_connected: BleStatusFacade[bool]
Is the camera connected to a PC via USB?
- video_hindsight: BleStatusFacade[bool]
Is Video Hindsight Capture Active?
- video_low_temp: BleStatusFacade[bool]
Is the camera getting too cold to continue recording?
- video_presets: BleStatusFacade[int]
Current Video Preset (ID).
- video_progress: BleStatusFacade[int]
When encoding video, this is the duration (seconds) of the video so far; 0 otherwise.
- video_rem: BleStatusFacade[int]
How many minutes of video can be captured with current settings before sdcard is full?
- wap_prov_stat: BleStatusFacade[PairType]
Wifi AP provisioning state.
- wap_scan_state: BleStatusFacade[PairType]
State of current scan for Wifi Access Points. Appears to only change for CAH-related scans.
- wifi_bars: BleStatusFacade[int]
Wifi signal strength in bars.
- wireless_band: BleStatusFacade[WifiBand]
Wireless Band.
- wireless_enabled: BleStatusFacade[bool]
Are Wireless Connections enabled?
- wlan_ssid: BleStatusFacade[str]
Provisioned WIFI AP SSID. On BLE connection, value is big-endian byte-encoded int.
- zoom_encoding: BleStatusFacade[bool]
Is this camera capable of zooming while encoding (static value based on model, not settings)?
- class HttpCommands(communicator: CommunicatorType)
Bases:
HttpMessages
[HttpMessage
]All of the HTTP commands.
To be used as a delegate for a GoProHttp to build commands
- async add_file_hilight(*, file: str, offset: int | None = None) GoProResp[None]
Add a hilight to a media file (.mp4)
- async delete_all() GoProResp[None]
Delete all files on the SD card.
- Returns:
command status
- Return type:
GoProResp[None]
- async delete_file(*, path: str) GoProResp[None]
Delete a single file including single files that are part of a group.
- async delete_group(*, path: str) GoProResp[None]
Delete all contents of a group. Should not be used on non-group files.
- async download_file(*, camera_file: str, local_file: Path | None = None) GoProResp[Path]
Download a video from the camera to a local file.
If local_file is none, the output location will be the same name as the camera_file.
- async get_camera_info() GoProResp[CameraInfo]
Get general information about the camera such as firmware version
- Returns:
status and settings as JSON
- Return type:
- async get_camera_state() GoProResp[dict[SettingId | StatusId, Any]]
Get all camera statuses and settings
- Returns:
status and settings as JSON
- Return type:
- async get_date_time() GoProResp[datetime]
Get the date and time of the camera (Non timezone / DST aware)
- Returns:
current date and time on camera
- Return type:
- async get_gpmf_data(*, camera_file: str, local_file: Path | None = None) GoProResp[Path]
Get GPMF data for a file.
If local_file is none, the output location will be the same name as the camera_file.
- async get_media_metadata(*, path: str) GoProResp[MediaMetadata]
Get media metadata for a file.
- Parameters:
path (str) – Path on camera of media file to get metadata for
- Returns:
Media metadata JSON structure
- Return type:
- async get_preset_status() GoProResp[dict[str, Any]]
Get status of current presets
- Returns:
JSON describing currently available presets and preset groups
- Return type:
GoProResp[JsonDict]
- async get_screennail__call__(*, camera_file: str, local_file: Path | None = None) GoProResp[Path]
Get screennail for a file.
If local_file is none, the output location will be the same name as the camera_file.
- async get_telemetry(*, camera_file: str, local_file: Path | None = None) GoProResp[Path]
Download the telemetry data for a camera file and store in a local file.
If local_file is none, the output location will be the same name as the camera_file.
- async get_thumbnail(*, camera_file: str, local_file: Path | None = None) GoProResp[Path]
Get thumbnail for a file.
If local_file is none, the output location will be the same name as the camera_file.
- async load_preset(*, preset: int) GoProResp[None]
Set camera to a given preset
The preset ID can be found from
open_gopro.api.http_commands.HttpCommands.get_preset_status
- async load_preset_group(*, group: int) GoProResp[None]
Set the active preset group.
The most recently used Preset in this group will be set.
- Parameters:
group (proto.EnumPresetGroup.ValueType) – desired Preset Group
- Returns:
command status
- Return type:
GoProResp[None]
- async remove_file_hilight(*, file: str, offset: int | None = None) GoProResp[None]
Remove a hilight from a media file (.mp4)
- async set_camera_control(*, mode: CameraControl) GoProResp[None]
Configure global behaviors by setting camera control (to i.e. Idle, External)
- Parameters:
mode (Params.CameraControl) – desired camera control value
- Returns:
command status
- Return type:
GoProResp[None]
- async set_date_time(*, date_time: datetime, tz_offset: int = 0, is_dst: bool = False) GoProResp[None]
Update the date and time of the camera
- Parameters:
date_time (datetime.datetime) – date and time
tz_offset (int) – timezone (as UTC offset). Defaults to 0.
is_dst (bool) – is daylight savings time?. Defaults to False.
- Returns:
command status
- Return type:
GoProResp[None]
- async set_keep_alive() GoProResp[None]
Send the keep alive signal to maintain the connection.
- Returns:
command status
- Return type:
GoProResp[None]
- async set_preview_stream(*, mode: Toggle, port: int | None = None) GoProResp[None]
Start or stop the preview stream
- Parameters:
mode (Params.Toggle) – enable to start or disable to stop
port (int | None) – Port to use for Preview Stream. Defaults to 8554 if None. Only relevant when starting the stream.
- Returns:
command status
- Return type:
GoProResp[None]
- async set_shutter(*, shutter: Toggle) GoProResp[None]
Set the shutter on or off
- Parameters:
shutter (Params.Toggle) – on or off (i.e. start or stop encoding)
- Returns:
command status
- Return type:
GoProResp[None]
- async set_third_party_client_info() GoProResp[None]
Flag as third party app
- Returns:
command status
- Return type:
GoProResp[None]
- async set_turbo_mode(*, mode: Toggle) GoProResp[None]
Enable or disable Turbo transfer mode.
- Parameters:
mode (Params.Toggle) – enable / disable turbo mode
- Returns:
Status
- Return type:
GoProResp[None]
- async update_custom_preset(*, icon_id: int | None = None, title_id: str | int | None = None, custom_name: str | None = None) GoProResp[None]
For a custom preset, update the Icon and / or the Title
- Parameters:
icon_id (proto.EnumPresetIcon.ValueType | None) – Icon to use. Defaults to None.
title_id (str | proto.EnumPresetTitle.ValueType | None) – Title to use. Defaults to None.
custom_name (str | None) – Custom name to use if title_id is set to proto.EnumPresetTitle.PRESET_TITLE_USER_DEFINED_CUSTOM_NAME. Defaults to None.
- Returns:
command status
- Return type:
GoProResp[None]
- async webcam_exit() GoProResp[WebcamResponse]
Exit the webcam.
- Returns:
command status
- Return type:
- async webcam_preview() GoProResp[WebcamResponse]
Start the webcam preview.
- Returns:
command status
- Return type:
- async webcam_start(*, resolution: WebcamResolution | None = None, fov: WebcamFOV | None = None, port: int | None = None, protocol: WebcamProtocol | None = None) GoProResp[WebcamResponse]
Start the webcam.
- Parameters:
resolution (Params.WebcamResolution | None) – resolution to use. If not set, camera default will be used.
fov (Params.WebcamFOV | None) – field of view to use. If not set, camera default will be used.
port (int | None) – port to use for streaming. If not set, camera default of 8554 will be used.
protocol (Params.WebcamProtocol | None) – streaming protocol to use. If not set, camera default of TS will be used.
- Returns:
command status
- Return type:
- async webcam_status() GoProResp[WebcamResponse]
Get the current status of the webcam
- Returns:
command status including the webcam status
- Return type:
- async webcam_stop() GoProResp[WebcamResponse]
Stop the webcam.
- Returns:
command status
- Return type:
- class HttpSettings(communicator: GoProHttp)
Bases:
HttpMessages
[HttpSetting
]The collection of all HTTP Settings
- Parameters:
communicator (GoProHttp) – Adapter to read / write settings
- anti_flicker: HttpSetting[AntiFlicker]
Anti Flicker frequency.
- auto_off: HttpSetting[AutoOff]
Set the auto off time.
- bit_depth: HttpSetting[BitDepth]
System Video Bit depth.
- bit_rate: HttpSetting[BitRate]
System Video Bit Rate.
- camera_ux_mode: HttpSetting[CameraUxMode]
Camera controls configuration.
- fps: HttpSetting[FPS]
Frames per second.
- framing: HttpSetting[Framing]
Video Framing Mode
- hindsight: HttpSetting[Hindsight]
Hindsight time / disable
- hypersmooth: HttpSetting[HypersmoothMode]
Set / disable hypersmooth.
- max_lens_mode: HttpSetting[MaxLensMode]
Enable / disable max lens mod.
- maxlens_mod_type: HttpSetting[MaxLensModType]
Max lens mod? If so, what type?
- maxlens_status: HttpSetting[Toggle]
Enable / disable max lens mod
- media_format: HttpSetting[MediaFormat]
Set the media format.
- multi_shot_easy_aspect_ratio: HttpSetting[EasyAspectRatio]
Multi shot easy aspect ratio
- multi_shot_field_of_view: HttpSetting[MultishotFOV]
Multi-shot FOV.
- multi_shot_nlv_aspect_ratio: HttpSetting[EasyAspectRatio]
Multi shot NLV aspect ratio
- photo_duration: HttpSetting[PhotoDuration]
Interval between photo captures
- photo_easy_mode: HttpSetting[PhotoEasyMode]
Night Photo easy mode.
- photo_field_of_view: HttpSetting[PhotoFOV]
Photo FOV.
- photo_horizon_leveling: HttpSetting[HorizonLeveling]
Lock / unlock horizon leveling for photo.
- photo_interval: HttpSetting[PhotoInterval]
Interval between photo captures
- photo_mode: HttpSetting[PhotoMode]
Photo Mode
- photo_output: HttpSetting[PhotoOutput]
File type of photo output
- resolution: HttpSetting[Resolution]
Resolution.
- star_trail_length: HttpSetting[StarTrailLength]
Multi shot star trail length.
- system_video_mode: HttpSetting[SystemVideoMode]
System video mode.
- timelapse_mode: HttpSetting[TimelapseMode]
Timelapse Mode
- video_aspect_ratio: HttpSetting[VideoAspectRatio]
Video aspect ratio
- video_duration: HttpSetting[VideoDuration]
If set, a video will automatically be stopped after recording for this long.
- video_easy_aspect_ratio: HttpSetting[EasyAspectRatio]
Video easy aspect ratio
- video_easy_mode: HttpSetting[int]
Video easy mode speed.
- video_field_of_view: HttpSetting[VideoFOV]
Video FOV.
- video_horizon_leveling: HttpSetting[HorizonLeveling]
Lock / unlock horizon leveling for video.
- video_mode: HttpSetting[VideoMode]
Video Mode (i.e. quality)
- video_performance_mode: HttpSetting[PerformanceMode]
Video Performance Mode (extended battery, tripod, etc).
- video_profile: HttpSetting[VideoProfile]
Video Profile (hdr, etc.)
- wifi_band: HttpSetting[WifiBand]
Current WiFi band being used.
Base Types
Commonly reused type aliases
- CmdType
Types that identify a command.
alias of
CmdId
|QueryCmdId
|ActionId
- ProducerType
Types that can be registered for.
alias of
tuple
[QueryCmdId
,SettingId
|StatusId
]
- ResponseType
Types that are used to identify a response.
alias of
CmdId
|QueryCmdId
|ActionId
|StatusId
|SettingId
|BleUUID
|str
|Enum
- UpdateCb
Callback definition for update handlers
alias of
Callable
[[SettingId
|StatusId
|ActionId
,Any
],Coroutine
[Any
,Any
,None
]]
GoPro Enum
- class GoProEnum(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- class GoProIntEnum(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
BLE Setting
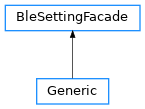
- class BleSettingFacade(communicator: GoProBle, identifier: SettingId, parser_builder: Construct | type[GoProIntEnum] | BytesParserBuilder)
Wrapper around BleSetting since a BleSetting’s message definition changes based on how it is being operated on.
- Raises:
TypeError – Parser builder is not a valid type
- Parameters:
- class BleSettingMessageBase(uuid: BleUUID, identifier: SettingId | QueryCmdId, setting_id: SettingId, builder: BuilderProtocol)
Actual BLE Setting Message that is wrapped by the facade.
- Parameters:
uuid (BleUUID) – UUID to access this setting.
identifier (SettingId | QueryCmdId) – How responses to operations on this message will be identified.
setting_id (SettingId) – Setting identifier. May match identifier in some cases.
builder (BuilderProtocol) – Build request bytes from the current message.
- async get_capabilities_values() GoProResp[list[ValueType]]
Get currently supported settings capabilities values.
- async get_value() GoProResp[ValueType]
Get the settings value.
- Returns:
settings value
- Return type:
GoProResp[ValueType]
- async register_capability_update(callback: Callable[[SettingId | StatusId | ActionId, Any], Coroutine[Any, Any, None]]) GoProResp[None]
Register for asynchronous notifications when a given setting ID’s capabilities update.
- async register_value_update(callback: Callable[[SettingId | StatusId | ActionId, Any], Coroutine[Any, Any, None]]) GoProResp[None]
Register for asynchronous notifications when a given setting ID’s value updates.
- async set(value: ValueType) GoProResp[None]
Set the value of the setting.
- Parameters:
value (ValueType) – The argument to use to set the setting value.
- Returns:
Status of set
- Return type:
GoProResp[None]
- async unregister_capability_update(callback: Callable[[SettingId | StatusId | ActionId, Any], Coroutine[Any, Any, None]]) GoProResp[None]
Stop receiving notifications when a given setting ID’s capabilities change.
BLE Status
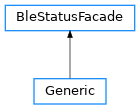
- class BleStatusFacade(communicator: GoProBle, identifier: StatusId, parser: Construct | type[GoProIntEnum] | BytesParserBuilder)
Wrapper around BleStatus since a BleStatus’s message definition changes based on how it is being operated on.
- Parameters:
- Raises:
TypeError – Attempted to pass an invalid parser type
- class BleStatusMessageBase(uuid: BleUUID, identifier: StatusId | QueryCmdId, status_id: StatusId, builder: Callable[[Any], bytearray])
An individual camera status that is interacted with via BLE.
- Parameters:
uuid (BleUUID) – UUID to access this status.
identifier (StatusId | QueryCmdId) – How responses to operations on this message will be identified.
status_id (StatusId) – Status identifier. May match identifier in some cases.
builder (Callable[[Any], bytearray]) – Build request bytes from the current message.
- async get_value() GoProResp[ValueType]
Get the current value of a status.
- Returns:
current status value
- Return type:
GoProResp[ValueType]
- async register_value_update(callback: Callable[[SettingId | StatusId | ActionId, Any], Coroutine[Any, Any, None]]) GoProResp[ValueType]
Register for asynchronous notifications when a status changes.
HTTP Setting
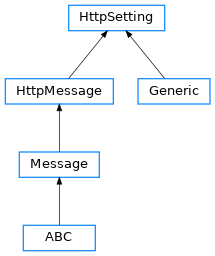
Method Protocols
- class BuilderProtocol(*args, **kwargs)
Protocol definition of data building methods
Message Bases
These are the base types that are used to implement version-specific API’s. These are published for reference but the end user should never need to use these directly.
- class Message(identifier: SettingId | StatusId | ActionId | CmdId | BleUUID | str, parser: Parser | None = None)
Bases:
ABC
Base class for all messages that will be contained in a Messages class
- class HttpMessage(endpoint: str, identifier: SettingId | StatusId | ActionId | CmdId | BleUUID | str | None, components: list[str] | None = None, arguments: list[str] | None = None, body_args: list[str] | None = None, headers: dict[str, Any] | None = None, certificate: Path | None = None, parser: Parser | None = None)
Bases:
Message
The base class for all HTTP messages. Stores common information.
- Parameters:
endpoint (str) – base endpoint
identifier (IdType | None) – explicit message identifier. If None, will be generated from endpoint.
components (list[str] | None) – Additional path components (i.e. endpoint/{COMPONENT}). Defaults to None.
arguments (list[str] | None) – Any arguments to be appended after endpoint (i.e. endpoint?{ARGUMENT}). Defaults to None.
body_args (list[str] | None) – Arguments to be added to the body JSON. Defaults to None.
headers (dict[str, Any] | None) – A dict of values to be set in the HTTP operation headers. Defaults to None.
certificate (Path | None) – Path to SSL certificate bundle. Defaults to None.
parser (Parser | None) – Parser to interpret HTTP responses. Defaults to None.
- class BleMessage(uuid: BleUUID, identifier: SettingId | StatusId | ActionId | CmdId | BleUUID | str, parser: Parser | None)
Bases:
Message
The base class for all BLE messages to store common info
- class Messages(communicator: CommunicatorType)
Bases:
ABC
,dict
,Generic
[MessageType
,CommunicatorType
]Base class for setting and status containers
Allows message groups to be iterable and supports dict-like access.
Instance attributes that are an instance (or subclass) of Message are automatically accumulated during instantiation
- Parameters:
communicator (CommunicatorType) – communicator that will send messages
- class BleMessages(communicator: CommunicatorType)
Bases:
Messages
[MessageType
,GoProBle
]A container of BLE Messages.
Identical to Messages and it just used for typing
- class HttpMessages(communicator: CommunicatorType)
Bases:
Messages
[MessageType
,GoProHttp
]A container of HTTP Messages.
Identical to Messages and it just used for typing
- class MessageRules(fastpass_analyzer: Analyzer = <function MessageRules.<lambda>>, wait_for_encoding_analyzer: Analyzer = <function MessageRules.<lambda>>)
Message Rules Manager
- Parameters:
- class Analyzer(*args, **kwargs)
Protocol definition of message rules analyzer
Parameters
All of these parameters can be accessed via:
from open_gopro import Params
Parameter definitions for GoPro BLE and WiFi commands for Open GoPro version 2_0
- class AccMicStatus(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- CONNECTED = 1
- CONNECTED_AND_PLUGGED_IN = 2
- NOT_CONNECTED = 0
- class AnalyticsState(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- NOT_READY = 0
- ON_CONNECT = 2
- READY = 1
- class AntiFlicker(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- HZ_50 = 3
- HZ_60 = 2
- class AutoOff(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- MIN_1 = 1
- MIN_15 = 6
- MIN_30 = 7
- MIN_5 = 4
- NEVER = 0
- SEC_30 = 12
- SEC_8 = 11
- class AutoPowerOff(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- MIN_1 = 1
- MIN_15 = 6
- MIN_30 = 7
- MIN_5 = 4
- NEVER = 0
- class BitDepth(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- BIT_10 = 2
- BIT_8 = 0
- class BitRate(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- HIGH = 1
- STANDARD = 0
- class CameraControl(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- CAMERA = 1
- EXTERNAL = 2
- IDLE = 0
- class CameraUxMode(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- EASY = 0
- PRO = 1
- class EasyAspectRatio(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- MOBILE = 1
- UNIVERSAL = 2
- WIDESCREEN = 0
- class ExposureMode(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- AUTO = 1
- DISABLED = 0
- HEMISPHERE = 3
- ISO_LOCK = 2
- class FPS(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- FPS_100 = 2
- FPS_120 = 1
- FPS_200 = 13
- FPS_24 = 10
- FPS_240 = 0
- FPS_25 = 9
- FPS_30 = 8
- FPS_50 = 6
- FPS_60 = 5
- class Flatmode(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- BURST_PHOTO = 19
- LIVE_BURST = 25
- LOOPING = 15
- NIGHT_LAPSE_PHOTO = 21
- NIGHT_LAPSE_VIDEO = 26
- NIGHT_PHOTO = 18
- NOT_APPLICABLE = 0
- SINGLE_PHOTO = 16
- SLO_MO = 27
- TIME_LAPSE_PHOTO = 20
- TIME_LAPSE_VIDEO = 13
- TIME_WARP_VIDEO = 24
- UNKNOWN = 28
- VIDEO = 12
- WEBCAM = 23
- class FrameRate(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- FRAME_RATE_100_0 = 2
- FRAME_RATE_120_0 = 1
- FRAME_RATE_200_0 = 13
- FRAME_RATE_240_0 = 0
- FRAME_RATE_24_0 = 10
- FRAME_RATE_25_0 = 9
- FRAME_RATE_300_0 = 17
- FRAME_RATE_30_0 = 8
- FRAME_RATE_360_0 = 16
- FRAME_RATE_400_0 = 15
- FRAME_RATE_50_0 = 6
- FRAME_RATE_60_0 = 5
- FRAME_RATE_90_0 = 3
- class Framing(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- FULL = 2
- VERTICAL = 1
- WIDESCREEN = 0
- class Hindsight(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- OFF = 4
- SEC_15 = 2
- SEC_30 = 3
- class HorizonLeveling(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- LOCKED = 2
- OFF = 0
- ON = 1
- class HypersmoothMode(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- AUTO_BOOST = 4
- BOOST = 3
- HIGH = 2
- OFF = 0
- ON = 1
- STANDARD = 100
- class LED(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- ALL_OFF = 4
- ALL_ON = 3
- BLE_KEEP_ALIVE = 66
- FRONT_OFF_ONLY = 5
- LED_2 = 2
- class LensAttachment(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- AUTO_DETECT = 100
- MACRO = 4
- MAX_LENS_2_0 = 2
- MAX_LENS_2_5 = 3
- ND_16 = 8
- ND_32 = 9
- ND_4 = 6
- ND_8 = 7
- STANDARD_LENS = 10
- class LensModStatus(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- ANAMORPHIC = 5
- DEFAULT = 0
- INVALID = -1
- MACRO = 4
- MAX_LENS = 1
- MAX_LENS_2_0 = 2
- MAX_LENS_2_5 = 3
- ND_16 = 8
- ND_32 = 9
- ND_4 = 6
- ND_8 = 7
- NONE = 10
- class LensMode(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- DUAL = 5
- SINGLE = 0
- class MaxLensModType(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- NONE = 0
- V1 = 1
- V2 = 2
- class MaxLensMode(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- DEFAULT = 0
- MAX_LENS = 1
- class MediaFormat(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- NIGHT_LAPSE_PHOTO = 21
- NIGHT_LAPSE_VIDEO = 26
- TIME_LAPSE_PHOTO = 20
- TIME_LAPSE_VIDEO = 13
- class MediaModMicStatus(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- ONLY = 1
- REMOVED = 0
- WITH_EXTERNAL = 2
- class MediaModStatus(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- SELFIE_0_HDMI_0_MEDIAMODCONNECTED_FALSE = 0
- SELFIE_0_HDMI_0_MEDIAMODCONNECTED_TRUE = 1
- SELFIE_0_HDMI_1_MEDIAMODCONNECTED_FALSE = 2
- SELFIE_0_HDMI_1_MEDIAMODCONNECTED_TRUE = 3
- SELFIE_1_HDMI_0_MEDIAMODCONNECTED_FALSE = 4
- SELFIE_1_HDMI_0_MEDIAMODCONNECTED_TRUE = 5
- SELFIE_1_HDMI_1_MEDIAMODCONNECTED_FALSE = 6
- SELFIE_1_HDMI_1_MEDIAMODCONNECTED_TRUE = 7
- class MultishotFOV(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- LINEAR = 102
- MAX_SUPERVIEW = 100
- NARROW = 19
- NOT_APPLICABLE = 0
- WIDE = 101
- class MultishotFraming(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- FRAMING_16_9 = 1
- FRAMING_4_3 = 0
- FRAMING_8_7 = 3
- FRAMING_9_16 = 4
- class OTAStatus(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- DOWNLOADING = 1
- DOWNLOAD_FAILED = 3
- GOPRO_APP_DOWNLOADING = 6
- GOPRO_APP_DOWNLOAD_FAILED = 8
- GOPRO_APP_READY = 10
- GOPRO_APP_VERIFYING = 7
- GOPRO_APP_VERIFY_FAILED = 9
- IDLE = 0
- READY = 5
- VERIFYING = 2
- VERIFY_FAILED = 4
- class Orientation(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- ON_LEFT_SIDE = 3
- ON_RIGHT_SIDE = 2
- UPRIGHT = 0
- UPSIDE_DOWN = 1
- class PairState(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- COMPLETED = 4
- FAILED = 2
- IN_PROGRESS = 1
- NOT_STARTED = 0
- STOPPED = 3
- class PairType(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- NOT_PAIRING = 0
- PAIRING_APP = 1
- PAIRING_BLUETOOTH = 3
- PAIRING_REMOTE_CONTROL = 2
- class PerformanceMode(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- EXTENDED_BATTERY = 1
- MAX_PERFORMANCE = 0
- STATIONARY = 2
- class PhotoDuration(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- HOUR_1 = 7
- HOUR_2 = 8
- HOUR_3 = 9
- MIN_1 = 3
- MIN_15 = 5
- MIN_30 = 6
- MIN_5 = 4
- OFF = 0
- SEC_15 = 1
- SEC_30 = 2
- class PhotoEasyMode(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- NIGHT_PHOTO = 101
- OFF = 0
- ON = 1
- SUPER_PHOTO = 100
- class PhotoFOV(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- HYPERVIEW = 9
- LINEAR = 102
- LINEAR_HORIZON = 121
- MAX_SUPERVIEW = 100
- NARROW = 19
- NOT_APPLICABLE = 0
- WIDE = 101
- class PhotoInterval(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- OFF = 0
- SEC_0_5 = 2
- SEC_1 = 3
- SEC_10 = 6
- SEC_120 = 9
- SEC_2 = 4
- SEC_3 = 10
- SEC_30 = 7
- SEC_5 = 5
- SEC_60 = 8
- class PhotoLensV2(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- MP_12_LINEAR = 10
- MP_12_ULTRA_WIDE = 41
- MP_12_WIDE = 0
- MP_13_LINEAR = 38
- MP_13_ULTRA_WIDE = 40
- MP_13_WIDE = 39
- MP_23_LINEAR = 28
- MP_23_WIDE = 27
- MP_27_LINEAR = 32
- MP_27_WIDE = 31
- MP_9_LINEAR = 37
- MP_9_WIDE = 15
- class PhotoMode(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- NIGHT = 1
- SUPER = 0
- class PhotoModeV2(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- BURST = 2
- NIGHT = 1
- SUPER = 0
- class PhotoOutput(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- HDR = 2
- RAW = 1
- STANDARD = 0
- SUPERPHOTO = 3
- class PresetGroup(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- PHOTO = 1001
- TIMELAPSE = 1002
- VIDEO = 1000
- class QualityControl(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- BASIC = 2
- HIGHEST = 0
- STANDARD = 1
- class RegionalFormat(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- HZ_50 = 1
- HZ_60 = 0
- class Resolution(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- NOT_APPLICABLE = 0
- RES_1080 = 9
- RES_1080_16_9 = 106
- RES_1080_8_7 = 110
- RES_1080_9_16 = 30
- RES_1440 = 7
- RES_2_7K = 4
- RES_2_7K_16_9 = 104
- RES_2_7K_4_3 = 6
- RES_2_7K_4_3_TODO = 105
- RES_2_7_K_8_7 = 11
- RES_4K = 1
- RES_4K_16_9 = 102
- RES_4K_4_3 = 18
- RES_4K_4_3_TODO = 103
- RES_4K_8_7 = 108
- RES_4K_8_7_ = 109
- RES_4K_8_7_LEGACY = 28
- RES_4K_9_16 = 29
- RES_5K = 24
- RES_5K_4_3 = 25
- RES_5_3K = 100
- RES_5_3K_16_9 = 101
- RES_5_3K_4_3 = 27
- RES_5_3K_8_7 = 107
- RES_5_3K_8_7_LEGACY = 26
- class SDStatus(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- BUSY = 4
- FORMAT_ERROR = 3
- FULL = 1
- OK = 0
- REMOVED = 2
- SWAPPED = 8
- UNKNOWN = 255
- class ScreenSaverTimeout(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- MIN_1 = 1
- MIN_2 = 2
- MIN_3 = 3
- MIN_5 = 4
- NEVER = 0
- class SetupLanguage(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- CHINESE = 8
- ENGLISH_AUS = 2
- ENGLISH_IND = 13
- ENGLISH_UK = 1
- ENGLISH_US = 0
- FRENCH = 4
- GERMAN = 3
- ITALIAN = 5
- JAPANESE = 9
- KOREAN = 10
- PORTUGUESE = 11
- RUSSIAN = 12
- SPANISH = 6
- SPANISH_NA = 7
- SWEDISH = 14
- class StarTrailLength(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- LONG = 2
- MAX = 3
- NOT_APPLICABLE = 0
- SHORT = 1
- class SystemVideoMode(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- EXTENDED_BATTERY = 1
- EXTENDED_BATTERY_GREEN_ICON = 101
- HIGHEST_QUALITY = 0
- LONGEST_BATTERY_GREEN_ICON = 102
- class TimeWarpSpeed(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- SPEED_10X = 9
- SPEED_150X = 3
- SPEED_15X = 0
- SPEED_1800X = 6
- SPEED_1X = 11
- SPEED_2X = 7
- SPEED_300X = 4
- SPEED_30X = 1
- SPEED_5X = 8
- SPEED_60X = 2
- SPEED_900X = 5
- SPEED_AUTO = 10
- SPEED_HALF = 12
- class TimelapseLensV2(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- MP_27_LINEAR = 32
- MP_27_WIDE = 31
- class TimelapseMode(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- LIGHT_PAINTING = 2
- MAX_LIGHT_PAINTING = 6
- MAX_STAR_TRAILS = 5
- MAX_TIMEWARP = 4
- MAX_VEHICLE_LIGHTS = 7
- STAR_TRAILS = 1
- TIMEWARP = 0
- VEHICLE_LIGHTS = 3
- class Toggle(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- DISABLE = 0
- ENABLE = 1
- class VideoAspectRatio(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- RATIO_16_9 = 1
- RATIO_4_3 = 0
- RATIO_8_7 = 3
- RATIO_9_16 = 4
- class VideoDuration(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- DUR_15_MINUTES = 5
- DUR_15_SECONDS = 1
- DUR_1_HOUR = 7
- DUR_1_MINUTE = 3
- DUR_2_HOURS = 8
- DUR_30_MINUTES = 6
- DUR_30_SECONDS = 2
- DUR_3_HOURS = 9
- DUR_5_MINUTES = 4
- DUR_5_SECONDS = 10
- DUR_NO_LIMIT = 100
- class VideoFOV(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- HYPERVIEW = 9
- LINEAR = 4
- LINEAR_HORIZON_LEVELING = 8
- LINEAR_HORIZON_LOCK = 10
- MAX_HYPERVIEW = 11
- MAX_SUPERVIEW = 7
- NARROW = 2
- SUPERVIEW = 3
- WIDE = 0
- class VideoFraming(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- FRAMING_16_9 = 1
- FRAMING_1_1 = 6
- FRAMING_4_3 = 0
- FRAMING_8_7 = 3
- FRAMING_9_16 = 4
- class VideoLensV2(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- HYPERVIEW = 9
- LINEAR = 4
- LINEAR_HORIZON_LEVELING = 8
- LINEAR_HORIZON_LOCK = 10
- MAX_HYPERVIEW = 11
- NARROW = 2
- SUPERVIEW = 3
- ULTRA_HYPERVIEW = 100
- ULTRA_SUPERVIEW = 12
- ULTRA_WIDE = 13
- WIDE = 0
- class VideoMode(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- BASIC = 2
- HIGHEST = 0
- STANDARD = 1
- class VideoProfile(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- HDR = 1
- LOG = 2
- STANDARD = 0
- class Volume(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- HIGH = 100
- LOW = 70
- MEDIUM = 85
- class WAPState(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- ABORTED = 2
- CANCELED = 3
- COMPLETED = 4
- NEVER_STARTED = 0
- STARTED = 1
- class WebcamFOV(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- LINEAR = 4
- NARROW = 2
- SUPERVIEW = 3
- WIDE = 0
- class WebcamProtocol(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- RTSP = 'RTSP'
- TS = 'TS'
Responses
Generic common response container:
This can be imported via:
from open_gopro import GoProResp
- class GoProResp(protocol: Protocol, status: ErrorCode, data: T, identifier: CmdId | QueryCmdId | ActionId | StatusId | SettingId | BleUUID | str | Enum)
The object used to encapsulate all GoPro responses.
It consists of several common properties / attribute and a data attribute that varies per response.
>>> gopro = WirelessGoPro() >>> await gopro.open() >>> response = await (gopro.ble_setting.resolution).get_value() >>> print(response)
Now let’s inspect the responses various attributes / properties:
>>> print(response.status) ErrorCode.SUCCESS >>> print(response.ok) True >>> print(response.identifier) QueryCmdId.GET_SETTING_VAL >>> print(response.protocol) Protocol.BLE
Now let’s print it’s data as (as JSON):
>>> print(response) { "id" : "QueryCmdId.GET_SETTING_VAL", "status" : "ErrorCode.SUCCESS", "protocol" : "Protocol.BLE", "data" : { "SettingId.RESOLUTION" : "Resolution.RES_4K_16_9", }, }
- protocol
protocol response was received on
- Type:
- data
parsed response data
- Type:
T
- identifier
response identifier, the type of which will vary depending on the response
- Type:
- class Protocol(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
Protocol that Command will be sent on.
Data Models
These are the various models that are returned in responses, used in commands, etc. They can be imported with:
from open_gopro.models import ***
- pydantic model MediaPath
Model to represent media path
- Fields:
- pydantic model MediaMetadata
Base Media Metadata class
- Fields:
- classmethod from_json(json_str: dict[str, Any]) MediaMetadata
Build a metadata object given JSON input
- Parameters:
json_str (JsonDict) – raw JSON
- Returns:
parsed metadata
- Return type:
- pydantic model PhotoMetadata
Bases:
MediaMetadata
Metadata for a Photo file
- pydantic model VideoMetadata
Bases:
MediaMetadata
Metadata for a video file
- Fields:
- field hilight_list: list[str] [Required] (alias 'hi')
List of hlights in ms offset from start of video
- pydantic model MediaItem
Base Media Item class
- Fields:
- pydantic model GroupedMediaItem
Bases:
MediaItem
Media Item that is also a grouped item.
An example of a grouped item is a burst photo.
- Fields:
- pydantic model MediaFileSystem
Grouping of media items into filesystem(s)
- pydantic model MediaList
Top level media list object
- field media: list[MediaFileSystem] [Required]
Media filesystem(s)
- pydantic model TzDstDateTime
DST aware datetime
- field datetime: datetime.datetime [Required]
- pydantic model CameraInfo
General camera info
- Config:
protected_namespaces: tuple = ()
- Fields:
- pydantic model WebcamResponse
Common Response from Webcam Commands
- Fields:
- field error: constants.WebcamError [Required]
- field status: constants.WebcamStatus | None = None
- field supported_options: list[SupportedOption] | None = None
Constants
These can be imported as:
from open_gopro import constants
Constant numbers shared across the GoPro module. These do not change across Open GoPro Versions
- class ActionId(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- GET_AP_ENTRIES = 3
- GET_AP_ENTRIES_RSP = 131
- GET_LIVESTREAM_STATUS = 116
- GET_PRESET_STATUS = 114
- GET_PRESET_STATUS_RSP = 242
- LIVESTREAM_STATUS_NOTIF = 245
- LIVESTREAM_STATUS_RSP = 244
- NOTIF_PROVIS_STATE = 12
- NOTIF_START_SCAN = 11
- PRESET_MODIFIED_NOTIFICATION = 243
- RELEASE_NETWORK = 120
- RELEASE_NETWORK_RSP = 248
- REQUEST_CLEAR_COHN_CERT = 102
- REQUEST_COHN_SETTING = 101
- REQUEST_CREATE_COHN_CERT = 103
- REQUEST_GET_COHN_CERT = 110
- REQUEST_GET_COHN_STATUS = 111
- REQUEST_GET_LAST_MEDIA = 109
- REQUEST_PRESET_UPDATE_CUSTOM = 100
- REQUEST_WIFI_CONNECT = 4
- REQUEST_WIFI_CONNECT_NEW = 5
- REQUEST_WIFI_CONNECT_NEW_RSP = 133
- REQUEST_WIFI_CONNECT_RSP = 132
- RESPONSE_CLEAR_COHN_CERT = 230
- RESPONSE_COHN_SETTING = 229
- RESPONSE_CREATE_COHN_CERT = 231
- RESPONSE_GET_COHN_CERT = 238
- RESPONSE_GET_COHN_STATUS = 239
- RESPONSE_GET_LAST_MEDIA = 237
- RESPONSE_PRESET_UPDATE_CUSTOM = 228
- SCAN_WIFI_NETWORKS = 2
- SCAN_WIFI_NETWORKS_RSP = 130
- SET_CAMERA_CONTROL = 105
- SET_CAMERA_CONTROL_RSP = 233
- SET_LIVESTREAM_MODE = 121
- SET_LIVESTREAM_MODE_RSP = 249
- SET_TURBO_MODE = 107
- SET_TURBO_MODE_RSP = 235
- class CmdId(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- GET_CAMERA_CAPABILITIES = 50
- GET_CAMERA_SETTINGS = 18
- GET_CAMERA_STATUSES = 19
- GET_DATE_TIME = 14
- GET_DATE_TIME_DST = 16
- GET_HW_INFO = 60
- GET_SETTINGS_JSON = 59
- GET_THIRD_PARTY_API_VERSION = 81
- LOAD_PRESET = 64
- LOAD_PRESET_GROUP = 62
- POWER_DOWN = 4
- REGISTER_ALL_CAPABILITIES = 98
- REGISTER_ALL_SETTINGS = 82
- REGISTER_ALL_STATUSES = 83
- SET_DATE_TIME = 13
- SET_DATE_TIME_DST = 15
- SET_PAIRING_COMPLETE = 3
- SET_SHUTTER = 1
- SET_THIRD_PARTY_CLIENT_INFO = 80
- SET_WIFI = 23
- SLEEP = 5
- TAG_HILIGHT = 24
- UNREGISTER_ALL_CAPABILITIES = 130
- UNREGISTER_ALL_SETTINGS = 114
- UNREGISTER_ALL_STATUSES = 115
- class ErrorCode(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- ERROR = 1
- INVALID_PARAM = 2
- SUCCESS = 0
- UNKNOWN = -1
- class FeatureId(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- COMMAND = 241
- NETWORK_MANAGEMENT = 2
- QUERY = 245
- SETTING = 243
- class GoProUUIDs(*_: Any)
GoPro Proprietary BleUUID’s.
- CM_NET_MGMT_COMM = Camera Management
- CN_NET_MGMT_RESP = Camera Management Response
- CQ_COMMAND = Command
- CQ_COMMAND_RESP = Command Response
- CQ_QUERY = Query
- CQ_QUERY_RESP = Query Response
- CQ_SENSOR = Sensor
- CQ_SENSOR_RESP = Sensor Response
- CQ_SETTINGS = Settings
- CQ_SETTINGS_RESP = Settings Response
- S_CAMERA_MANAGEMENT = Camera Management Service
- S_CONTROL_QUERY = Control and Query Service
- S_WIFI_ACCESS_POINT = Wifi Access Point Service
- WAP_CSI_PASSWORD = CSI Password
- WAP_PASSWORD = Wifi AP Password
- WAP_POWER = Wifi Power
- WAP_SSID = Wifi AP SSID
- WAP_STATE = Wifi State
- class QueryCmdId(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- GET_CAPABILITIES_NAME = 66
- GET_CAPABILITIES_VAL = 50
- GET_SETTING_NAME = 34
- GET_SETTING_VAL = 18
- GET_STATUS_VAL = 19
- PROTOBUF_QUERY = 245
- REG_CAPABILITIES_UPDATE = 98
- REG_SETTING_VAL_UPDATE = 82
- REG_STATUS_VAL_UPDATE = 83
- SETTING_CAPABILITY_PUSH = 162
- SETTING_VAL_PUSH = 146
- STATUS_VAL_PUSH = 147
- UNREG_CAPABILITIES_UPDATE = 130
- UNREG_SETTING_VAL_UPDATE = 114
- UNREG_STATUS_VAL_UPDATE = 115
- class SettingId(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- ADDON_MAX_LENS_MOD = 189
- ADDON_MAX_LENS_MOD_ENABLE = 190
- ANTI_FLICKER = 134
- AUTO_OFF = 59
- AUTO_POWER_OFF = 225
- BIT_DEPTH = 183
- BIT_RATE = 182
- CAMERA_UX_MODE = 175
- CAMERA_VOLUME = 216
- FPS = 3
- FRAME_RATE = 234
- FRAMING = 193
- HINDSIGHT = 167
- HYPERSMOOTH = 135
- LED = 91
- LENS_ATTACHMENT = 217
- MAX_LENS_MOD = 162
- MEDIA_FORMAT = 128
- MULTI_SHOT_EASY_ASPECT_RATIO = 188
- MULTI_SHOT_FOV = 123
- MULTI_SHOT_FRAMING = 233
- MULTI_SHOT_NLV_ASPECT_RATIO = 192
- PHOTO_DIGITAL_LENSES_V2 = 230
- PHOTO_EASY_MODE = 177
- PHOTO_FOV = 122
- PHOTO_HORIZON_LEVELING = 151
- PHOTO_INTERVAL = 171
- PHOTO_INTERVAL_DURATION = 172
- PHOTO_MODE = 191
- PHOTO_MODE_V2 = 227
- PHOTO_OUTPUT = 125
- PROTOBUF_SETTING = 243
- QUALITY_CONTROL = 201
- REGIONAL_FORMAT = 195
- RESOLUTION = 2
- SETUP_LANGUAGE = 223
- SETUP_SCREEN_SAVER = 219
- STAR_TRAIL_LENGTH = 179
- SYSTEM_VIDEO_MODE = 180
- TIMELAPSE_DIGITAL_LENSES_V2 = 231
- TIMELAPSE_MODE = 187
- VIDEO_ASPECT_RATIO = 108
- VIDEO_DIGITAL_LENSES_V2 = 229
- VIDEO_DURATION = 156
- VIDEO_EASY_ASPECT_RATIO = 185
- VIDEO_EASY_MODE = 176
- VIDEO_FOV = 121
- VIDEO_FRAMING = 232
- VIDEO_HORIZON_LEVELING = 150
- VIDEO_MODE = 186
- VIDEO_PERFORMANCE_MODE = 173
- VIDEO_PROFILE = 184
- WIFI_BAND = 178
- class StatusId(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
- ACC_MIC_STAT = 74
- ACTIVE_PRESET = 97
- ANALYTICS_RDY = 61
- ANALYTICS_SIZE = 62
- APP_COUNT = 31
- AP_SSID = 30
- AP_STATE = 69
- BAND_5GHZ_AVAIL = 81
- BATT_LEVEL = 2
- BATT_OK_OTA = 83
- BATT_PRESENT = 1
- CAMERA_CONTROL = 114
- CAMERA_LENS_MOD = 119
- CAMERA_LENS_TYPE = 105
- CAPTURE_DELAY = 84
- CAPT_DELAY_ACTIVE = 101
- CONTROL_OVER_USB = 116
- CREATING_PRESET = 109
- CURRENT_TIME_MS = 57
- DIGITAL_ZOOM = 75
- DIG_ZOOM_ACTIVE = 77
- DOWNLOAD_CANCEL_PEND = 42
- ENCODING = 10
- EXPOSURE_TYPE = 65
- EXPOSURE_X = 66
- EXPOSURE_Y = 67
- FIRST_TIME = 79
- FLATMODE_ID = 89
- GPS_STAT = 68
- INT_BATT_PER = 70
- IN_CONTEXT_MENU = 63
- LAST_HILIGHT = 59
- LCD_LOCK_ACTIVE = 11
- LINUX_CORE_ACTIVE = 104
- LIVE_BURST_REM = 99
- LIVE_BURST_TOTAL = 100
- LOCATE_ACTIVE = 45
- LOGS_READY = 91
- MEDIA_MOD_MIC_STAT = 102
- MEDIA_MOD_STAT = 110
- MOBILE_VIDEO = 78
- MODE_GROUP = 43
- MULTI_COUNT_DOWN = 49
- NEXT_POLL = 60
- NUM_GROUP_PHOTO = 36
- NUM_GROUP_VIDEO = 37
- NUM_HILIGHTS = 58
- NUM_TOTAL_PHOTO = 38
- NUM_TOTAL_VIDEO = 39
- ORIENTATION = 86
- OTA_STAT = 41
- PAIR_STATE = 19
- PAIR_STATE2 = 28
- PAIR_TIME = 21
- PAIR_TYPE = 20
- PHOTOS_REM = 34
- PHOTO_INTERVAL_CAPTURE_COUNT = 118
- PHOTO_PRESETS = 94
- PRESETS_GROUP = 96
- PRESET_MODIFIED = 98
- PREVIEW_ENABLED = 32
- QUICK_CAPTURE = 9
- REMOTE_CTRL_CONN = 27
- REMOTE_CTRL_VER = 26
- SCHEDULED_CAPTURE = 108
- SCHEDULED_PRESET = 107
- SD_RATING_CHECK_ERROR = 111
- SD_STATUS = 33
- SD_WRITE_SPEED_ERROR = 112
- SEC_SD_STAT = 80
- SPACE_REM = 54
- STREAMING_SUPP = 55
- SYSTEM_BUSY = 8
- SYSTEM_HOT = 6
- SYSTEM_READY = 82
- TIMELAPSE_PRESETS = 95
- TIMELAPSE_REM = 64
- TIMEWARP_SPEED_RAMP = 103
- TOTAL_SD_SPACE_KB = 117
- TURBO_MODE = 113
- USB_CONNECTED = 115
- VIDEO_HINDSIGHT = 106
- VIDEO_LOW_TEMP = 85
- VIDEO_PRESETS = 93
- VIDEO_PROGRESS = 13
- VIDEO_REM = 35
- WAP_PROV_STAT = 24
- WAP_SCAN_STATE = 22
- WAP_SCAN_TIME = 23
- WIFI_BARS = 56
- WIRELESS_BAND = 76
- WIRELESS_ENABLED = 17
- WLAN_SSID = 29
- ZOOM_ENCODING = 88
Exceptions
Exceptions that pertain to Gopro-level functionality.
- exception ConnectFailed(connection: str, timeout: float, retries: int)
A BLE connection failed to establish
- exception ConnectionTerminated(message: str)
A connection that was previously established has terminated.
- exception FailedToFindDevice
The scan failed without finding a device.
- exception GoProError
Base class for other GoPro-level exceptions.
- exception GoProNotOpened(message: str)
A command was attempted without waiting for the GoPro instance to open.
- exception InterfaceConfigFailure
An error has occurred while setting up the communication interface
- exception InvalidConfiguration(message: str)
Something was attempted that is not possible for the current configuration.
- exception InvalidOpenGoProVersion(version: str)
Attempt to access an invalid Open GoPro API version
Common Interface
Parser Protocol and Bases
- class BaseParser
Base Parser Interface
A parser is something that transforms input into a different type
- abstract parse(data: T) T_co
Parse data into output type
- Parameters:
data (T) – input data to parse
- Returns:
parsed output
- Return type:
T_co
- class BaseTransformer
Transformer interface.
A transformer is something that transforms the input into the same output type
- abstract transform(data: T) T
Transform data into output matching the input type
- Parameters:
data (T) – data to transform
- Returns:
transformed data
- Return type:
T
- class BytesBuilder(*args, **kwargs)
Base bytes serializer protocol definition
- class BytesParser
Bytes to Target Type Parser Interface
- class BytesParserBuilder(*args, **kwargs)
Class capable of both building / parsing bytes to / from object
- class BytesTransformer
Bytes to Bytes transformer interface
- class GlobalParsers
Parsers that relate globally to ID’s as opposed to contextualized per-message
This is intended to be used as a singleton, i.e. not instantiated
- classmethod add(identifier: CmdId | QueryCmdId | ActionId | StatusId | SettingId | BleUUID | str | Enum, parser: Parser) None
Add a global parser that can be accessed by this class’s class methods
- Parameters:
identifier (ResponseType) – identifier to add parser for
parser (Parser) – parser to add
- classmethod add_feature_action_id_mapping(feature_id: FeatureId, action_id: ActionId) None
Add a feature id-to-action id mapping entry
- classmethod get_parser(identifier: CmdId | QueryCmdId | ActionId | StatusId | SettingId | BleUUID | str | Enum) Parser | None
Get a globally defined parser for the given ID.
Currently, only BLE uses globally defined parsers
- Parameters:
identifier (ResponseType) – ID to get parser for
- Returns:
parser if found, else None
- Return type:
Parser | None
- classmethod get_query_container(identifier: CmdId | QueryCmdId | ActionId | StatusId | SettingId | BleUUID | str | Enum) Callable | None
Attempt to get a callable that will translate an input value to the ID-appropriate value.
For example, _get_query_container(SettingId.RESOLUTION) will return
open_gopro.api.params.Resolution
As another example, _get_query_container(StatusId.TURBO_MODE) will return bool()
Note! Not all ID’s are currently parsed so None will be returned if the container does not exist
- Parameters:
identifier (ResponseType) – identifier to find container for
- Returns:
container if found else None
- Return type:
Callable | None
- class JsonParser
Json to Target Type Parser Interface
- class JsonTransformer
Json to json transformer interface
- class Parser(byte_transformers: list[BytesTransformer] | None = None, byte_json_adapter: BytesParser[dict[str, Any]] | None = None, json_transformers: list[JsonTransformer] | None = None, json_parser: JsonParser[T] | None = None)
The common monolithic Parser that is used for all byte and json parsing / transforming
- Algorithm is:
Variable number of byte transformers (bytes –> bytes)
One bytes Json adapter (bytes –> json)
Variable number of json transformers (json –> json)
One JSON parser (json -> Any)
- Parameters:
byte_transformers (list[BytesTransformer] | None) – bytes –> bytes. Defaults to None.
byte_json_adapter (BytesParser[JsonDict] | None) – bytes –> json. Defaults to None.
json_transformers (list[JsonTransformer] | None) – json –> json. Defaults to None.
json_parser (JsonParser[T] | None) – json –> T. Defaults to None.
- parse(data: bytes | bytearray | dict[str, Any]) T
Perform the parsing using the stored transformers and parsers
- Parameters:
data (bytes | bytearray | JsonDict) – input bytes or json to parse
- Raises:
RuntimeError – attempted to parse bytes when a byte-json adapter does not exist
- Returns:
final parsed output
- Return type:
T
- class GoProBase(**kwargs: Any)
The base class for communicating with all GoPro Clients
- abstract property ble_command: BleCommands
Used to call the BLE commands
- Returns:
the commands
- Return type:
- abstract property ble_setting: BleSettings
Used to access the BLE settings
- Returns:
the settings
- Return type:
- abstract property ble_status: BleStatuses
Used to access the BLE statuses
- Returns:
the statuses
- Return type:
- abstract async configure_cohn(timeout: int = 60) bool
Prepare Camera on the Home Network
Provision if not provisioned Then wait for COHN to be connected and ready
- abstract property http_command: HttpCommands
Used to access the Wifi commands
- Returns:
the commands
- Return type:
- abstract property http_setting: HttpSettings
Used to access the Wifi settings
- Returns:
the settings
- Return type:
- abstract property identifier: str
Unique identifier for the connected GoPro Client
- Returns:
identifier
- Return type:
- abstract property is_ble_connected: bool
Are we connected via BLE to the GoPro device?
- Returns:
True if yes, False if no
- Return type:
- abstract property is_cohn_provisioned: bool
Is COHN currently provisioned?
Get the current COHN status from the camera
- Returns:
True if COHN is provisioned, False otherwise
- Return type:
- abstract property is_http_connected: bool
Are we connected via HTTP to the GoPro device?
- Returns:
True if yes, False if no
- Return type:
- abstract property is_open: bool
Is this client ready for communication?
- Returns:
True if yes, False if no
- Return type:
- abstract property is_ready: bool
Is gopro ready to receive commands
- Returns:
yes if ready, no otherwise
- Return type:
- class GoProBle(controller: BLEController, disconnected_cb: Callable[[BleDevice], None], notification_cb: Callable[[int, bytearray], None], target: Pattern | BleDevice)
GoPro specific BLE Client
- Parameters:
controller (BLEController) – controller implementation to use for this client
disconnected_cb (DisconnectHandlerType) – disconnected callback
notification_cb (NotiHandlerType) – notification callback
target (Pattern | BleDevice) – regex or device to connect to
- class GoProHttp
Interface definition for all HTTP communicators
- class GoProWifi(controller: WifiController)
GoPro specific WiFi Client
- Parameters:
controller (WifiController) – instance of Wifi Controller to use for this client
- class GoProWiredInterface
The top-level interface for a Wired Open GoPro controller
- class GoProWirelessInterface(ble_controller: BLEController, wifi_controller: WifiController | None, disconnected_cb: Callable[[BleDevice], None], notification_cb: Callable[[int, bytearray], None], target: Pattern | BleDevice)
The top-level interface for a Wireless Open GoPro controller
This always supports BLE and can optionally support Wifi
- Parameters:
ble_controller (BLEController) – BLE controller instance
wifi_controller (WifiController | None) – Wifi controller instance
disconnected_cb (DisconnectHandlerType) – callback for BLE disconnects
notification_cb (NotiHandlerType) – callback for BLE received notifications
target (Pattern | BleDevice) – BLE device to search for
- class BaseGoProCommunicator
Common Communicator interface
- abstract register_update(callback: Callable[[SettingId | StatusId | ActionId, Any], Coroutine[Any, Any, None]], update: SettingId | StatusId | ActionId) None
Register for callbacks when an update occurs
- Parameters:
callback (UpdateCb) – callback to be notified in
update (UpdateType) – update to register for
- abstract unregister_update(callback: Callable[[SettingId | StatusId | ActionId, Any], Coroutine[Any, Any, None]], update: SettingId | StatusId | ActionId | None = None) None
Unregister for asynchronous update(s)
- Parameters:
callback (UpdateCb) – callback to stop receiving update(s) on
update (UpdateType | None) – updates to unsubscribe for. Defaults to None (all updates that use this callback will be unsubscribed).
BLE Interface
BLE Controller Interface Definition.
- class BLEController(exception_handler: Callable | None = None)
Interface definition for a BLE driver to be used by GoPro.
- abstract async connect(disconnect_cb: Callable[[BleDevice], None], device: BleDevice, timeout: int = 15) BleHandle
Connect to a BLE device.
- Parameters:
disconnect_cb (DisconnectHandlerType) – function to call when disconnect is received
device (BleDevice) – device to connect to
timeout (int) – How long to attempt connecting before giving up. Defaults to 15.
- Returns:
handle that has been connected to
- Return type:
BleHandle
- abstract async disconnect(handle: BleHandle) None
Terminate the BLE connection.
- Parameters:
handle (BleHandle) – handle to disconnect from
- abstract async discover_chars(handle: BleHandle, uuids: type[UUIDs] | None = None) GattDB
Discover all characteristics for a connected handle.
By default, the BLE controller only knows Spec-Defined BleUUID’s so any additional BleUUID’s should be passed in with the uuids argument
- abstract async enable_notifications(handle: BleHandle, handler: Callable[[int, bytearray], None]) None
Enable notifications for all notifiable characteristics.
The handler is used to register for notifications. It will be called when a a notification is received.
- Parameters:
handle (BleHandle) – handle to enable notifications on
handler (NotiHandlerType) – notification handler
- abstract async pair(handle: BleHandle) None
Pair to an already connected handle.
- Parameters:
handle (BleHandle) – handle to pair to
- abstract async read(handle: BleHandle, uuid: BleUUID) bytes
Read a bytestream response from a BleUUID.
Generic BLE Client definition that is composed of a BLE Controller.
- class BleClient(controller: BLEController, disconnected_cb: Callable[[BleDevice], None], notification_cb: Callable[[int, bytearray], None], target: tuple[Pattern | BleDevice, list[BleUUID] | None], uuids: type[UUIDs] | None = None)
A BLE device that is to be connected to.
- Parameters:
controller (BLEController) – controller implementation to use for this client
disconnected_cb (DisconnectHandlerType) – disconnected callback
notification_cb (NotiHandlerType) – notification callback
target (tuple[Pattern | BleDevice, list[BleUUID] | None]) – Tuple of: (device, service_uuids) where device is the BLE device (or regex) to connect to and service_uuids is a list of service uuid’s to filter for
uuids (type[UUIDs] | None) – Additional UUIDs that will be used when discovering characteristic. Defaults to None in which case any unknown UUIDs will be set to “unknown”.
- Raises:
ValueError – Must pass a valid target
- property gatt_db: GattDB
Return the attribute table
- Raises:
RuntimeError – GATT table hasn’t been discovered
- Returns:
table of BLE attributes
- Return type:
- property identifier: str | None
A string that identifies the GoPro
- Returns:
identifier or None if client has not yet been discovered
- Return type:
Optional[str]
- property is_connected: bool
Is BLE currently connected?
- Returns:
True if yes, False if no
- Return type:
- property is_discovered: bool
Has the target been discovered (via BLE scanning)?
- Returns:
True if yes, False if no
- Return type:
- async open(timeout: int = 10, retries: int = 5) None
Open the client resource so that it is ready to send and receive data.
- Parameters:
- Raises:
ConnectFailed – The BLE connection was not able to establish
BLEServices
Objects to nicely interact with BLE services, characteristics, and attributes.
- class BleUUID(name: str, format: Format = Format.BIT_128, hex: str | None = None, bytes: bytes | None = None, bytes_le: bytes | None = None, int: int | None = None)
An extension of the standard UUID to associate a string name with the UUID and allow 8-bit UUID input
Can only be initialized with one of [hex, bytes, bytes_le, int]
- Parameters:
name (str) – human readable name
format (BleUUID.Format) – 16 or 128 bit format. Defaults to BleUUID.Format.BIT_128.
hex (str | None) – build from hex string. Defaults to None.
bytes (bytes | None) – build from big-endian bytes. Defaults to None.
bytes_le (bytes | None) – build from little-endian bytes. Defaults to None.
int (int | None) – build from int. Defaults to None.
- Raises:
ValueError – Attempt to initialize with more than one option
ValueError – Badly formed input
- class Format(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
Used to specify 8-bit or 128-bit UUIDs
- class CharProps(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
BLE Spec-Defined Characteristic Property bitmask values
- class Characteristic(handle: int, uuid: BleUUID, props: CharProps, value: bytes | None = None, init_descriptors: dataclasses.InitVar[list[open_gopro.ble.services.Descriptor] | None] = None, descriptor_handle: int | None = None)
A BLE characteristic.
- Parameters:
handle (int) – the handle of the attribute table that the characteristic resides at
uuid (BleUUID) – the BleUUID of the characteristic
props (CharProps) – the characteristic’s properties (READ, WRITE, NOTIFY, etc)
value (bytes | None) – the current byte stream value of the characteristic value
init_descriptors (list[Descriptor] | None) – Descriptors known at initialization (can also be set later using the descriptors property)
descriptor_handle (int | None) – handle of this characteristic’s declaration descriptor. If not passed, defaults to handle + 1
- property cccd_handle: int
What is this characteristics CCCD (client characteristic configuration descriptor) handle
- Returns:
the CCCD handle
- Return type:
- property descriptors: dict[BleUUID, Descriptor]
Return uuid-to-descriptor mapping
- Returns:
dictionary of descriptors indexed by BleUUID
- Return type:
- property is_indicatable: bool
Does this characteristic have indicatable property?
- Returns:
True if indicatable, False if not
- Return type:
- property is_notifiable: bool
Does this characteristic have notifiable property?
- Returns:
True if notifiable, False if not
- Return type:
- property is_readable: bool
Does this characteristic have readable property?
- Returns:
True if readable, False if not
- Return type:
- property is_writeable: bool
Does this characteristic have writeable property?
That is, does it have writeable-with-response or writeable-without-response property
- Returns:
True if writeable, False if not
- Return type:
- property is_writeable_with_response: bool
Does this characteristic have writeable-with-response property?
- Returns:
True if writeable-with-response, False if not
- Return type:
- class Descriptor(handle: int, uuid: BleUUID, value: bytes | None = None)
A characteristic descriptor.
- Parameters:
- class GattDB(init_services: list[Service])
The attribute table to store / look up BLE services, characteristics, and attributes.
- Parameters:
init_services (list[Service]) – A list of services known at instantiation time. Can be updated later with the services property
- class CharacteristicView(db: GattDB)
Represent the GattDB mapping as characteristics indexed by BleUUID
- items() Generator[tuple[BleUUID, Characteristic], None, None]
Generate dict-like items view
- Returns:
items generator
- Return type:
Generator[tuple[BleUUID, Characteristic], None, None]
- keys() Generator[BleUUID, None, None]
Generate dict-like keys view
- Returns:
keys generator
- Return type:
Generator[BleUUID, None, None]
- values() Generator[Characteristic, None, None]
Generate dict-like values view
- Returns:
values generator
- Return type:
Generator[Characteristic, None, None]
- dump_to_csv(file: Path = PosixPath('attributes.csv')) None
Dump discovered services to a csv file.
- Parameters:
file (Path) – File to write to. Defaults to “./attributes.csv”.
- class Service(uuid: BleUUID, start_handle: int, end_handle: int = 65535, init_chars: dataclasses.InitVar[Optional[list[open_gopro.ble.services.Characteristic]]] = None)
A BLE service or grouping of Characteristics.
- Parameters:
uuid (BleUUID) – the service’s BleUUID
start_handle (int) – the attribute handle where the service begins
end_handle (int) – the attribute handle where the service ends. Defaults to 0xFFFF.
init_chars (list[Characteristic]) – list of characteristics known at service instantiation. Can be set later with the characteristics property
- property characteristics: dict[BleUUID, Characteristic]
Return uuid-to-characteristic mapping
- Returns:
Dict of characteristics indexed by uuid
- Return type:
- class SpecUuidNumber(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
BLE Spec-Defined BleUUID Number values as ints
- class UUIDs(*_: Any)
BLE Spec-defined UUIDs that are common across all applications.
Also functions as a dict to look up UUID’s by str, int, or BleUUID
- class UUIDsMeta(name, bases, dct)
The metaclass used to build a UUIDs container
Upon creation of a new UUIDs class, this will store the BleUUID names in an internal mapping indexed by UUID as int
WiFi Interface
Wifi Controller Interface Definition.
- class SsidState(value, names=None, *, module=None, qualname=None, type=None, start=1, boundary=None)
Current state of the SSID
- class WifiController(interface: str | None = None, password: str | None = None)
Interface definition for a Wifi driver to be used by GoPro.
- Parameters:
- abstract disconnect() bool
Disconnect from a network.
- Returns:
True if successful, False otherwise
- Return type:
- abstract property is_on: bool
Is the wireless driver currently enabled.
- Returns:
True if yes. False if no.
- Return type:
Open GoPro WiFi Client Implementation
- class WifiClient(controller: WifiController)
A Wifi client that is composed of, among other things, a Wifi interface
The interface is generic and can be set with the ‘controller’ argument
- Parameters:
controller (WifiController) – controller implementation to use for this client
- property is_connected: bool
Is the WiFi connection currently established?
- Returns:
True if yes, False if no
- Return type: