Open GoPro Python SDK
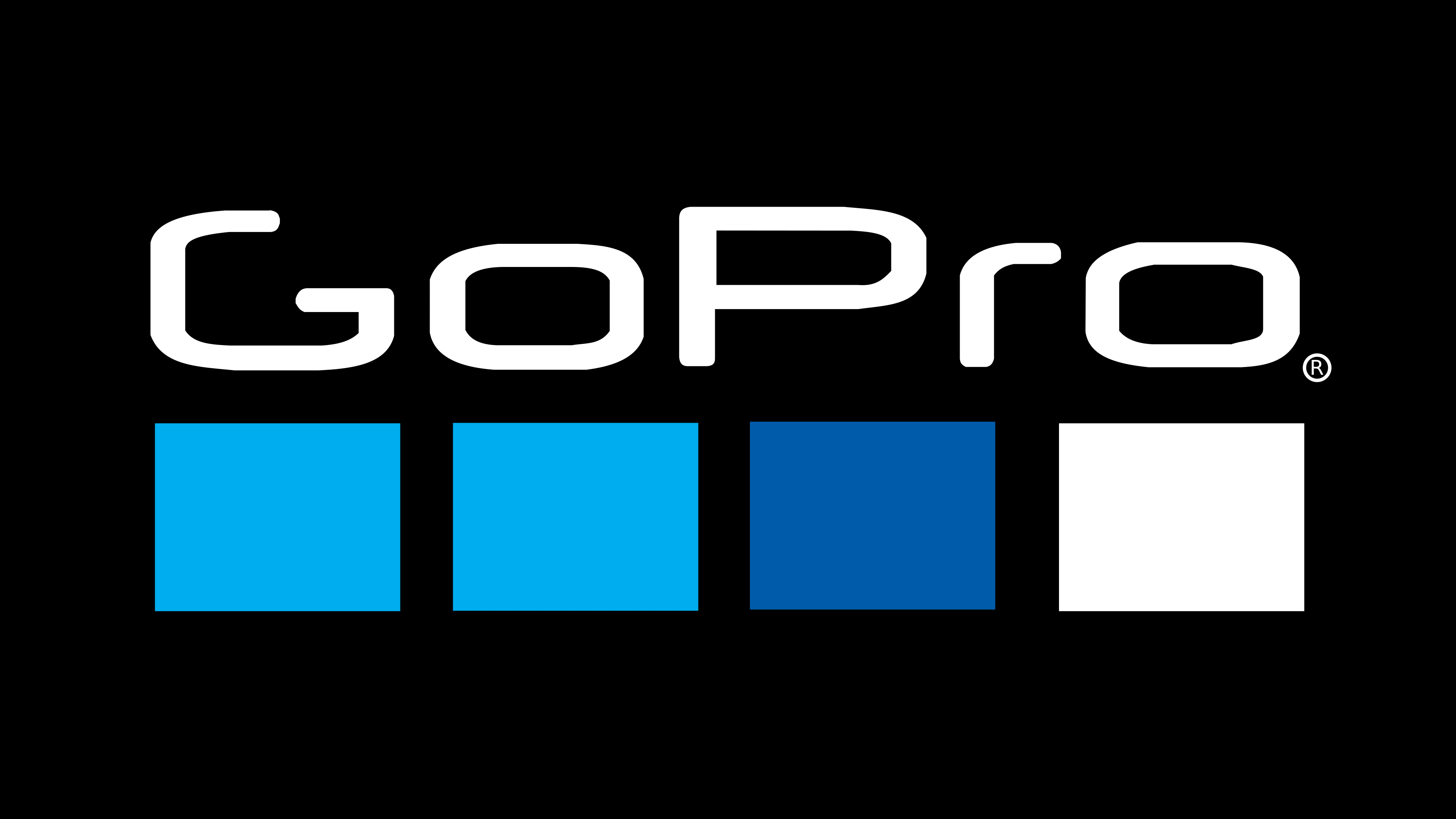
Summary
Welcome to the Open GoPro Python package documentation. This is a Python package that provides an interface for the user to exercise the Open GoPro Bluetooth Low Energy (BLE) and Wi-Fi / USB HTTP API’s as well as install command line interfaces to take photos, videos, and view video streams.
This package implements the API as defined in the Open GoPro Specification . For more information on the API, see the relevant documentation:
Warning
This package requires Python >= version 3.11 and < 3.14 and only supports GoPros that implement the OGP API
Features
Top-level GoPro class interface to use BLE, WiFi, and / or USB
Cross-platform (tested on Windows 10, and Ubuntu 20.04, and >= MacOS Big Sur)
BLE controller implemented using bleak
Wi-Fi controller provided in the Open GoPro package (loosely based on the Wireless Library )
Supports all wireless commands, settings, and statuses from the Open GoPro API
Automatically handles connection maintenance:
manage camera ready / encoding
periodically sends keep alive signals
Includes detailed logging for each module
Includes demo scripts installed as command-line applications to show BLE, WiFi, and USB functionality such as:
Take a photo
GUI to send all commands and view the live / preview stream
Configure and view a GoPro webcam stream
Take a video
Start a livestream
View the GoPro as a webcam
Log the battery
Getting Started
Here is a suggested procedure for getting acquainted with this package (it is the same as reading through this document in order):
Install the package
Try some of the demos
Implement your own example, perhaps starting with a demo, with usage information
If you need more detailed implementation reference, see the Interface documentation
Development
Set up the development environment
Open a Pull Request
Contents:
- Installation
- QuickStart Guide
- Usage
- API Reference
- GoPro Client
- Open GoPro API
BleCommands
BleCommands.cohn_clear_certificate()
BleCommands.cohn_create_certificate()
BleCommands.cohn_get_certificate()
BleCommands.cohn_get_status()
BleCommands.cohn_set_setting()
BleCommands.custom_preset_update()
BleCommands.enable_wifi_ap()
BleCommands.get_ap_entries()
BleCommands.get_camera_capabilities()
BleCommands.get_camera_settings()
BleCommands.get_camera_statuses()
BleCommands.get_date_time()
BleCommands.get_date_time_tz_dst()
BleCommands.get_hardware_info()
BleCommands.get_last_captured_media()
BleCommands.get_observable_for_all_capabilities()
BleCommands.get_observable_for_all_settings()
BleCommands.get_observable_for_all_statuses()
BleCommands.get_open_gopro_api_version()
BleCommands.get_preset_status()
BleCommands.get_wifi_password()
BleCommands.get_wifi_ssid()
BleCommands.load_preset()
BleCommands.load_preset_group()
BleCommands.power_down()
BleCommands.reboot()
BleCommands.register_livestream_status()
BleCommands.release_network()
BleCommands.request_wifi_connect()
BleCommands.request_wifi_connect_new()
BleCommands.scan_wifi_networks()
BleCommands.set_camera_control()
BleCommands.set_date_time()
BleCommands.set_date_time_tz_dst()
BleCommands.set_livestream_mode()
BleCommands.set_shutter()
BleCommands.set_third_party_client_info()
BleCommands.set_turbo_mode()
BleCommands.sleep()
BleCommands.tag_hilight()
BleSettings
BleSettings.anti_flicker
BleSettings.auto_power_down
BleSettings.bit_depth
BleSettings.camera_volume
BleSettings.control_mode
BleSettings.easy_mode_speed
BleSettings.easy_night_photo
BleSettings.enable_night_photo
BleSettings.frame_rate
BleSettings.frames_per_second
BleSettings.framing
BleSettings.gps
BleSettings.hindsight
BleSettings.hypersmooth
BleSettings.lapse_mode
BleSettings.lcd_brightness
BleSettings.led
BleSettings.max_lens
BleSettings.max_lens_mod
BleSettings.max_lens_mod_enable
BleSettings.media_format
BleSettings.multi_shot_aspect_ratio
BleSettings.multi_shot_duration
BleSettings.multi_shot_framing
BleSettings.nightlapse_rate
BleSettings.photo_horizon_leveling
BleSettings.photo_interval_duration
BleSettings.photo_lens
BleSettings.photo_mode
BleSettings.photo_output
BleSettings.photo_single_interval
BleSettings.photo_timelapse_rate
BleSettings.profiles
BleSettings.scheduled_capture
BleSettings.setup_language
BleSettings.setup_screen_saver
BleSettings.star_trails_length
BleSettings.system_video_mode
BleSettings.time_lapse_digital_lenses
BleSettings.video_aspect_ratio
BleSettings.video_bit_rate
BleSettings.video_duration
BleSettings.video_easy_mode
BleSettings.video_framing
BleSettings.video_horizon_leveling
BleSettings.video_lens
BleSettings.video_performance_mode
BleSettings.video_resolution
BleSettings.video_timelapse_rate
BleSettings.webcam_digital_lenses
BleSettings.wireless_band
BleStatuses
BleStatuses.access_point_ssid
BleStatuses.active_hilights
BleStatuses.ap_mode
BleStatuses.battery_present
BleStatuses.busy
BleStatuses.camera_control_id
BleStatuses.capture_delay_active
BleStatuses.cold
BleStatuses.connected_devices
BleStatuses.connected_wifi_ssid
BleStatuses.display_mod_status
BleStatuses.encoding
BleStatuses.flatmode
BleStatuses.ftu
BleStatuses.gps_lock
BleStatuses.hindsight
BleStatuses.last_pairing_success
BleStatuses.last_pairing_type
BleStatuses.last_wifi_scan_success
BleStatuses.lcd_lock
BleStatuses.lens_type
BleStatuses.linux_core
BleStatuses.live_bursts
BleStatuses.liveview_exposure_select_mode
BleStatuses.liveview_x
BleStatuses.liveview_y
BleStatuses.locate
BleStatuses.media_mod_state
BleStatuses.microphone_accessory
BleStatuses.minimum_status_poll_period
BleStatuses.mobile_friendly
BleStatuses.num_5ghz_available
BleStatuses.ota
BleStatuses.ota_charged
BleStatuses.overheating
BleStatuses.pairing_state
BleStatuses.pending_fw_update_cancel
BleStatuses.photo_interval_capture_count
BleStatuses.photo_preset
BleStatuses.photos
BleStatuses.preset
BleStatuses.preset_group
BleStatuses.preset_modified
BleStatuses.preview_stream
BleStatuses.preview_stream_available
BleStatuses.primary_storage
BleStatuses.quick_capture
BleStatuses.ready
BleStatuses.remaining_live_bursts
BleStatuses.remaining_photos
BleStatuses.remaining_video_time
BleStatuses.remote_connected
BleStatuses.remote_version
BleStatuses.rotation
BleStatuses.scheduled_capture
BleStatuses.scheduled_capture_preset_id
BleStatuses.sd_card_capacity
BleStatuses.sd_card_errors
BleStatuses.sd_card_remaining
BleStatuses.sd_card_write_speed_error
BleStatuses.time_since_last_hilight
BleStatuses.time_warp_speed
BleStatuses.timelapse_interval_countdown
BleStatuses.timelapse_preset
BleStatuses.turbo_transfer
BleStatuses.usb_connected
BleStatuses.usb_controlled
BleStatuses.video_encoding_duration
BleStatuses.video_preset
BleStatuses.videos
BleStatuses.wifi_bars
BleStatuses.wifi_provisioning_state
BleStatuses.wifi_scan_state
BleStatuses.wireless_band
BleStatuses.wireless_connections_enabled
BleStatuses.zoom_available
BleStatuses.zoom_level
BleStatuses.zoom_while_encoding
HttpCommands
HttpCommands.add_file_hilight()
HttpCommands.delete_all()
HttpCommands.delete_file()
HttpCommands.delete_group()
HttpCommands.download_file()
HttpCommands.get_camera_info()
HttpCommands.get_camera_state()
HttpCommands.get_date_time()
HttpCommands.get_gpmf_data()
HttpCommands.get_last_captured_media()
HttpCommands.get_media_list()
HttpCommands.get_media_metadata()
HttpCommands.get_open_gopro_api_version()
HttpCommands.get_preset_status()
HttpCommands.get_screennail()
HttpCommands.get_telemetry()
HttpCommands.get_thumbnail()
HttpCommands.get_webcam_version()
HttpCommands.load_preset()
HttpCommands.load_preset_group()
HttpCommands.reboot()
HttpCommands.remove_file_hilight()
HttpCommands.set_camera_control()
HttpCommands.set_date_time()
HttpCommands.set_digital_zoom()
HttpCommands.set_keep_alive()
HttpCommands.set_preview_stream()
HttpCommands.set_shutter()
HttpCommands.set_third_party_client_info()
HttpCommands.set_turbo_mode()
HttpCommands.update_custom_preset()
HttpCommands.webcam_exit()
HttpCommands.webcam_preview()
HttpCommands.webcam_start()
HttpCommands.webcam_status()
HttpCommands.webcam_stop()
HttpCommands.wired_usb_control()
HttpSettings
HttpSettings.anti_flicker
HttpSettings.auto_power_down
HttpSettings.bit_depth
HttpSettings.camera_volume
HttpSettings.control_mode
HttpSettings.easy_mode_speed
HttpSettings.easy_night_photo
HttpSettings.enable_night_photo
HttpSettings.frame_rate
HttpSettings.frames_per_second
HttpSettings.framing
HttpSettings.gps
HttpSettings.hindsight
HttpSettings.hypersmooth
HttpSettings.lapse_mode
HttpSettings.lcd_brightness
HttpSettings.led
HttpSettings.max_lens
HttpSettings.max_lens_mod
HttpSettings.max_lens_mod_enable
HttpSettings.media_format
HttpSettings.multi_shot_aspect_ratio
HttpSettings.multi_shot_duration
HttpSettings.multi_shot_framing
HttpSettings.nightlapse_rate
HttpSettings.photo_horizon_leveling
HttpSettings.photo_interval_duration
HttpSettings.photo_lens
HttpSettings.photo_mode
HttpSettings.photo_output
HttpSettings.photo_single_interval
HttpSettings.photo_timelapse_rate
HttpSettings.profiles
HttpSettings.scheduled_capture
HttpSettings.setup_language
HttpSettings.setup_screen_saver
HttpSettings.star_trails_length
HttpSettings.system_video_mode
HttpSettings.time_lapse_digital_lenses
HttpSettings.video_aspect_ratio
HttpSettings.video_bit_rate
HttpSettings.video_duration
HttpSettings.video_easy_mode
HttpSettings.video_framing
HttpSettings.video_horizon_leveling
HttpSettings.video_lens
HttpSettings.video_performance_mode
HttpSettings.video_resolution
HttpSettings.video_timelapse_rate
HttpSettings.webcam_digital_lenses
HttpSettings.wireless_band
- Abstracted Features
- Base Types
- Responses
- Constants
ActionId
ActionId.GET_AP_ENTRIES
ActionId.GET_AP_ENTRIES_RSP
ActionId.GET_LIVESTREAM_STATUS
ActionId.GET_PRESET_STATUS
ActionId.GET_PRESET_STATUS_RSP
ActionId.LIVESTREAM_STATUS_NOTIF
ActionId.LIVESTREAM_STATUS_RSP
ActionId.NOTIF_PROVIS_STATE
ActionId.NOTIF_START_SCAN
ActionId.PRESET_MODIFIED_NOTIFICATION
ActionId.RELEASE_NETWORK
ActionId.RELEASE_NETWORK_RSP
ActionId.REQUEST_CLEAR_COHN_CERT
ActionId.REQUEST_COHN_SETTING
ActionId.REQUEST_CREATE_COHN_CERT
ActionId.REQUEST_GET_COHN_CERT
ActionId.REQUEST_GET_COHN_STATUS
ActionId.REQUEST_GET_LAST_MEDIA
ActionId.REQUEST_PRESET_UPDATE_CUSTOM
ActionId.REQUEST_WIFI_CONNECT
ActionId.REQUEST_WIFI_CONNECT_NEW
ActionId.REQUEST_WIFI_CONNECT_NEW_RSP
ActionId.REQUEST_WIFI_CONNECT_RSP
ActionId.RESPONSE_CLEAR_COHN_CERT
ActionId.RESPONSE_COHN_SETTING
ActionId.RESPONSE_CREATE_COHN_CERT
ActionId.RESPONSE_GET_COHN_CERT
ActionId.RESPONSE_GET_COHN_STATUS
ActionId.RESPONSE_GET_LAST_MEDIA
ActionId.RESPONSE_PRESET_UPDATE_CUSTOM
ActionId.SCAN_WIFI_NETWORKS
ActionId.SCAN_WIFI_NETWORKS_RSP
ActionId.SET_CAMERA_CONTROL
ActionId.SET_CAMERA_CONTROL_RSP
ActionId.SET_LIVESTREAM_MODE
ActionId.SET_LIVESTREAM_MODE_RSP
ActionId.SET_TURBO_MODE
ActionId.SET_TURBO_MODE_RSP
CameraControl
CmdId
CmdId.GET_CAMERA_CAPABILITIES
CmdId.GET_CAMERA_SETTINGS
CmdId.GET_CAMERA_STATUSES
CmdId.GET_DATE_TIME
CmdId.GET_DATE_TIME_DST
CmdId.GET_HW_INFO
CmdId.GET_SETTINGS_JSON
CmdId.GET_THIRD_PARTY_API_VERSION
CmdId.LOAD_PRESET
CmdId.LOAD_PRESET_GROUP
CmdId.POWER_DOWN
CmdId.REBOOT
CmdId.REGISTER_ALL_CAPABILITIES
CmdId.REGISTER_ALL_SETTINGS
CmdId.REGISTER_ALL_STATUSES
CmdId.SET_DATE_TIME
CmdId.SET_DATE_TIME_DST
CmdId.SET_PAIRING_COMPLETE
CmdId.SET_SHUTTER
CmdId.SET_THIRD_PARTY_CLIENT_INFO
CmdId.SET_WIFI
CmdId.SLEEP
CmdId.TAG_HILIGHT
CmdId.UNREGISTER_ALL_CAPABILITIES
CmdId.UNREGISTER_ALL_SETTINGS
CmdId.UNREGISTER_ALL_STATUSES
ErrorCode
FeatureId
LED_SPECIAL
QueryCmdId
QueryCmdId.GET_CAPABILITIES_NAME
QueryCmdId.GET_CAPABILITIES_VAL
QueryCmdId.GET_SETTING_NAME
QueryCmdId.GET_SETTING_VAL
QueryCmdId.GET_STATUS_VAL
QueryCmdId.PROTOBUF_QUERY
QueryCmdId.REG_CAPABILITIES_UPDATE
QueryCmdId.REG_SETTING_VAL_UPDATE
QueryCmdId.REG_STATUS_VAL_UPDATE
QueryCmdId.SETTING_CAPABILITY_PUSH
QueryCmdId.SETTING_VAL_PUSH
QueryCmdId.STATUS_VAL_PUSH
QueryCmdId.UNREG_CAPABILITIES_UPDATE
QueryCmdId.UNREG_SETTING_VAL_UPDATE
QueryCmdId.UNREG_STATUS_VAL_UPDATE
Toggle
WebcamError
WebcamFOV
WebcamProtocol
WebcamResolution
WebcamStatus
Anti_Flicker
AutoPowerDown
BitDepth
CameraVolume
ControlMode
EasyModeSpeed
EasyModeSpeed.NUM_100_4X_SUPER_SLO_MO_SPEED_21_9_4K_V2_
EasyModeSpeed.NUM_100_4X_SUPER_SLO_MO_SPEED_2_7K_4_3_V2_
EasyModeSpeed.NUM_120_4X_SUPER_SLO_MO_SPEED_21_9_4K_V2_
EasyModeSpeed.NUM_120_4X_SUPER_SLO_MO_SPEED_2_7K_4_3_V2_
EasyModeSpeed.NUM_1X_NORMAL_SPEED_1_1_25_FPS_4K_V2_
EasyModeSpeed.NUM_1X_NORMAL_SPEED_1_1_30_FPS_4K_V2_
EasyModeSpeed.NUM_1X_NORMAL_SPEED_21_9_25_FPS_4K_V2_
EasyModeSpeed.NUM_1X_NORMAL_SPEED_21_9_25_FPS_5_3K_V2_
EasyModeSpeed.NUM_1X_NORMAL_SPEED_21_9_30_FPS_4K_V2_
EasyModeSpeed.NUM_1X_NORMAL_SPEED_21_9_30_FPS_5_3K_V2_
EasyModeSpeed.NUM_1X_NORMAL_SPEED_25_FPS_4_3_4K_V2_
EasyModeSpeed.NUM_1X_NORMAL_SPEED_25_FPS_4_3_5_3K_V2_
EasyModeSpeed.NUM_1X_NORMAL_SPEED_30_FPS_4_3_4K_V2_
EasyModeSpeed.NUM_1X_NORMAL_SPEED_30_FPS_4_3_5_3K_V2_
EasyModeSpeed.NUM_1X_SPEED_2_7K_50HZ_LOW_LIGHT_V2_
EasyModeSpeed.NUM_1X_SPEED_2_7K_LOW_LIGHT_V2_
EasyModeSpeed.NUM_1X_SPEED_4K_50HZ_FULL_FRAME_LOW_LIGHT_V2_
EasyModeSpeed.NUM_1X_SPEED_4K_50HZ_LOW_LIGHT_V2_
EasyModeSpeed.NUM_1X_SPEED_4K_FULL_FRAME_LOW_LIGHT_V2_
EasyModeSpeed.NUM_1X_SPEED_4K_LOW_LIGHT_V2_
EasyModeSpeed.NUM_1X_SPEED_50HZ_EXT_BATT_LOW_LIGHT_
EasyModeSpeed.NUM_1X_SPEED_50HZ_FULL_FRAME_LOW_LIGHT_V2_
EasyModeSpeed.NUM_1X_SPEED_50HZ_LONG_BATT_LOW_LIGHT_
EasyModeSpeed.NUM_1X_SPEED_50HZ_LONG_BATT_LOW_LIGHT_V2_
EasyModeSpeed.NUM_1X_SPEED_50HZ_LONG_BATT_LOW_LIGHT_V2_VERTICAL_
EasyModeSpeed.NUM_1X_SPEED_50HZ_LOW_LIGHT_
EasyModeSpeed.NUM_1X_SPEED_50HZ_LOW_LIGHT_V2_
EasyModeSpeed.NUM_1X_SPEED_50HZ_LOW_LIGHT_V2_VERTICAL_
EasyModeSpeed.NUM_1X_SPEED_EXT_BATT_LOW_LIGHT_
EasyModeSpeed.NUM_1X_SPEED_FULL_FRAME_LOW_LIGHT_V2_
EasyModeSpeed.NUM_1X_SPEED_LONG_BATT_LOW_LIGHT_
EasyModeSpeed.NUM_1X_SPEED_LONG_BATT_LOW_LIGHT_V2_
EasyModeSpeed.NUM_1X_SPEED_LONG_BATT_LOW_LIGHT_V2_VERTICAL_
EasyModeSpeed.NUM_1X_SPEED_LOW_LIGHT_
EasyModeSpeed.NUM_1X_SPEED_LOW_LIGHT_V2_
EasyModeSpeed.NUM_1X_SPEED_LOW_LIGHT_V2_VERTICAL_
EasyModeSpeed.NUM_2X_SLO_MO
EasyModeSpeed.NUM_2X_SLO_MO_2_7K_50HZ_V2_
EasyModeSpeed.NUM_2X_SLO_MO_2_7K_V2_
EasyModeSpeed.NUM_2X_SLO_MO_4K_
EasyModeSpeed.NUM_2X_SLO_MO_4K_50HZ_
EasyModeSpeed.NUM_2X_SLO_MO_4K_50HZ_V2_
EasyModeSpeed.NUM_2X_SLO_MO_4K_V2_
EasyModeSpeed.NUM_2X_SLO_MO_50HZ_
EasyModeSpeed.NUM_2X_SLO_MO_50HZ_EXT_BATT_
EasyModeSpeed.NUM_2X_SLO_MO_50HZ_FULL_FRAME_V2_
EasyModeSpeed.NUM_2X_SLO_MO_50HZ_LONG_BATT_
EasyModeSpeed.NUM_2X_SLO_MO_50HZ_LONG_BATT_V2_
EasyModeSpeed.NUM_2X_SLO_MO_50HZ_LONG_BATT_V2_VERTICAL_
EasyModeSpeed.NUM_2X_SLO_MO_50HZ_V2_
EasyModeSpeed.NUM_2X_SLO_MO_50HZ_V2_VERTICAL_
EasyModeSpeed.NUM_2X_SLO_MO_EXT_BATT_
EasyModeSpeed.NUM_2X_SLO_MO_FULL_FRAME_V2_
EasyModeSpeed.NUM_2X_SLO_MO_LONG_BATT_
EasyModeSpeed.NUM_2X_SLO_MO_LONG_BATT_V2_
EasyModeSpeed.NUM_2X_SLO_MO_LONG_BATT_V2_VERTICAL_
EasyModeSpeed.NUM_2X_SLO_MO_SPEED_1_1_4K_50_FPS_V2_
EasyModeSpeed.NUM_2X_SLO_MO_SPEED_1_1_4K_60_FPS_V2_
EasyModeSpeed.NUM_2X_SLO_MO_SPEED_21_9_4K_50_FPS_V2_
EasyModeSpeed.NUM_2X_SLO_MO_SPEED_21_9_4K_60_FPS_V2_
EasyModeSpeed.NUM_2X_SLO_MO_SPEED_21_9_5_3K_50_FPS_V2_
EasyModeSpeed.NUM_2X_SLO_MO_SPEED_21_9_5_3K_60_FPS_V2_
EasyModeSpeed.NUM_2X_SLO_MO_SPEED_4_3_4K_50_FPS_V2_
EasyModeSpeed.NUM_2X_SLO_MO_SPEED_4_3_4K_60_FPS_V2_
EasyModeSpeed.NUM_2X_SLO_MO_V2_
EasyModeSpeed.NUM_2X_SLO_MO_V2_VERTICAL_
EasyModeSpeed.NUM_4X_SUPER_SLO_MO
EasyModeSpeed.NUM_4X_SUPER_SLO_MO_2_7K_
EasyModeSpeed.NUM_4X_SUPER_SLO_MO_2_7K_50HZ_
EasyModeSpeed.NUM_4X_SUPER_SLO_MO_50HZ_
EasyModeSpeed.NUM_4X_SUPER_SLO_MO_50HZ_EXT_BATT_
EasyModeSpeed.NUM_4X_SUPER_SLO_MO_50HZ_LONG_BATT_
EasyModeSpeed.NUM_4X_SUPER_SLO_MO_50HZ_LONG_BATT_V2_
EasyModeSpeed.NUM_4X_SUPER_SLO_MO_50HZ_V2_
EasyModeSpeed.NUM_4X_SUPER_SLO_MO_EXT_BATT_
EasyModeSpeed.NUM_4X_SUPER_SLO_MO_LONG_BATT_
EasyModeSpeed.NUM_4X_SUPER_SLO_MO_LONG_BATT_V2_
EasyModeSpeed.NUM_4X_SUPER_SLO_MO_V2_
EasyModeSpeed.NUM_8X_ULTRA_SLO_MO
EasyModeSpeed.NUM_8X_ULTRA_SLO_MO_50HZ_
EasyModeSpeed.NUM_8X_ULTRA_SLO_MO_50HZ_EXT_BATT_
EasyModeSpeed.NUM_8X_ULTRA_SLO_MO_50HZ_LONG_BATT_
EasyModeSpeed.NUM_8X_ULTRA_SLO_MO_50HZ_LONG_BATT_V2_
EasyModeSpeed.NUM_8X_ULTRA_SLO_MO_50HZ_V2_
EasyModeSpeed.NUM_8X_ULTRA_SLO_MO_EXT_BATT_
EasyModeSpeed.NUM_8X_ULTRA_SLO_MO_LONG_BATT_
EasyModeSpeed.NUM_8X_ULTRA_SLO_MO_LONG_BATT_V2_
EasyModeSpeed.NUM_8X_ULTRA_SLO_MO_V2_
EasyNightPhoto
EnableNightPhoto
FrameRate
FramesPerSecond
FramesPerSecond.NUM_100_0
FramesPerSecond.NUM_120_0
FramesPerSecond.NUM_200_0
FramesPerSecond.NUM_240_0
FramesPerSecond.NUM_24_0
FramesPerSecond.NUM_25_0
FramesPerSecond.NUM_300_0
FramesPerSecond.NUM_30_0
FramesPerSecond.NUM_360_0
FramesPerSecond.NUM_400_0
FramesPerSecond.NUM_50_0
FramesPerSecond.NUM_60_0
Framing
Gps
Hindsight
Hypersmooth
LapseMode
Led
MaxLens
MaxLensMod
MaxLensModEnable
MediaFormat
MultiShotAspectRatio
MultiShotDuration
MultiShotDuration.NO_LIMIT
MultiShotDuration.NUM_15_MINUTES
MultiShotDuration.NUM_15_SECONDS
MultiShotDuration.NUM_1_HOUR
MultiShotDuration.NUM_1_MINUTE
MultiShotDuration.NUM_2_HOURS
MultiShotDuration.NUM_30_MINUTES
MultiShotDuration.NUM_30_SECONDS
MultiShotDuration.NUM_3_HOURS
MultiShotDuration.NUM_5_MINUTES
MultiShotFraming
NightlapseRate
NightlapseRate.AUTO
NightlapseRate.NUM_10_SECONDS
NightlapseRate.NUM_15_SECONDS
NightlapseRate.NUM_20_SECONDS
NightlapseRate.NUM_2_MINUTES
NightlapseRate.NUM_30_MINUTES
NightlapseRate.NUM_30_SECONDS
NightlapseRate.NUM_4_SECONDS
NightlapseRate.NUM_5_MINUTES
NightlapseRate.NUM_5_SECONDS
NightlapseRate.NUM_60_MINUTES
NightlapseRate.NUM_60_SECONDS
PhotoHorizonLeveling
PhotoIntervalDuration
PhotoIntervalDuration.NUM_15_MINUTES
PhotoIntervalDuration.NUM_15_SECONDS
PhotoIntervalDuration.NUM_1_HOUR
PhotoIntervalDuration.NUM_1_MINUTE
PhotoIntervalDuration.NUM_2_HOURS
PhotoIntervalDuration.NUM_30_MINUTES
PhotoIntervalDuration.NUM_30_SECONDS
PhotoIntervalDuration.NUM_3_HOURS
PhotoIntervalDuration.NUM_5_MINUTES
PhotoIntervalDuration.OFF
PhotoLens
PhotoLens.LINEAR
PhotoLens.LINEAR_12_MP
PhotoLens.LINEAR_23_MP
PhotoLens.LINEAR_27_MP
PhotoLens.MAX_SUPERVIEW
PhotoLens.NARROW
PhotoLens.NUM_13MP_LINEAR
PhotoLens.NUM_13MP_ULTRA_LINEAR
PhotoLens.NUM_13MP_ULTRA_WIDE
PhotoLens.NUM_13MP_WIDE
PhotoLens.ULTRA_WIDE_12_MP
PhotoLens.WIDE
PhotoLens.WIDE_12_MP
PhotoLens.WIDE_23_MP
PhotoLens.WIDE_27_MP
PhotoMode
PhotoOutput
PhotoSingleInterval
PhotoTimelapseRate
PhotoTimelapseRate.NUM_0_5_SECONDS
PhotoTimelapseRate.NUM_10_SECONDS
PhotoTimelapseRate.NUM_1_SECOND
PhotoTimelapseRate.NUM_2_MINUTES
PhotoTimelapseRate.NUM_2_SECONDS
PhotoTimelapseRate.NUM_30_MINUTES
PhotoTimelapseRate.NUM_30_SECONDS
PhotoTimelapseRate.NUM_3_SECONDS
PhotoTimelapseRate.NUM_5_MINUTES
PhotoTimelapseRate.NUM_5_SECONDS
PhotoTimelapseRate.NUM_60_MINUTES
PhotoTimelapseRate.NUM_60_SECONDS
Profiles
SettingId
SettingId.ANTI_FLICKER
SettingId.AUTO_POWER_DOWN
SettingId.BIT_DEPTH
SettingId.CAMERA_VOLUME
SettingId.CONTROL_MODE
SettingId.EASY_MODE_SPEED
SettingId.EASY_NIGHT_PHOTO
SettingId.ENABLE_NIGHT_PHOTO
SettingId.FRAMES_PER_SECOND
SettingId.FRAME_RATE
SettingId.FRAMING
SettingId.GPS
SettingId.HINDSIGHT
SettingId.HYPERSMOOTH
SettingId.LAPSE_MODE
SettingId.LCD_BRIGHTNESS
SettingId.LED
SettingId.MAX_LENS
SettingId.MAX_LENS_MOD
SettingId.MAX_LENS_MOD_ENABLE
SettingId.MEDIA_FORMAT
SettingId.MULTI_SHOT_ASPECT_RATIO
SettingId.MULTI_SHOT_DURATION
SettingId.MULTI_SHOT_FRAMING
SettingId.NIGHTLAPSE_RATE
SettingId.PHOTO_HORIZON_LEVELING
SettingId.PHOTO_INTERVAL_DURATION
SettingId.PHOTO_LENS
SettingId.PHOTO_MODE
SettingId.PHOTO_OUTPUT
SettingId.PHOTO_SINGLE_INTERVAL
SettingId.PHOTO_TIMELAPSE_RATE
SettingId.PROFILES
SettingId.SCHEDULED_CAPTURE
SettingId.SETUP_LANGUAGE
SettingId.SETUP_SCREEN_SAVER
SettingId.STAR_TRAILS_LENGTH
SettingId.SYSTEM_VIDEO_MODE
SettingId.TIME_LAPSE_DIGITAL_LENSES
SettingId.VIDEO_ASPECT_RATIO
SettingId.VIDEO_BIT_RATE
SettingId.VIDEO_DURATION
SettingId.VIDEO_EASY_MODE
SettingId.VIDEO_FRAMING
SettingId.VIDEO_HORIZON_LEVELING
SettingId.VIDEO_LENS
SettingId.VIDEO_PERFORMANCE_MODE
SettingId.VIDEO_RESOLUTION
SettingId.VIDEO_TIMELAPSE_RATE
SettingId.WEBCAM_DIGITAL_LENSES
SettingId.WIRELESS_BAND
SetupLanguage
SetupLanguage.CHINESE
SetupLanguage.ENGLISH_AUS
SetupLanguage.ENGLISH_IND
SetupLanguage.ENGLISH_UK
SetupLanguage.ENGLISH_US
SetupLanguage.FRENCH
SetupLanguage.GERMAN
SetupLanguage.ITALIAN
SetupLanguage.JAPANESE
SetupLanguage.KOREAN
SetupLanguage.PORTUGUESE
SetupLanguage.RUSSIAN
SetupLanguage.SPANISH
SetupLanguage.SPANISH_NA
SetupLanguage.SWEDISH
SetupScreenSaver
StarTrailsLength
SystemVideoMode
TimeLapseDigitalLenses
VideoAspectRatio
VideoBitRate
VideoDuration
VideoEasyMode
VideoFraming
VideoHorizonLeveling
VideoLens
VideoPerformanceMode
VideoResolution
VideoResolution.NUM_1080
VideoResolution.NUM_1080_9_16_V2
VideoResolution.NUM_1440
VideoResolution.NUM_2_7K
VideoResolution.NUM_2_7K_4_3
VideoResolution.NUM_2_7K_4_3_V2
VideoResolution.NUM_4K
VideoResolution.NUM_4K_1_1
VideoResolution.NUM_4K_21_9
VideoResolution.NUM_4K_4_3
VideoResolution.NUM_4K_4_3_V2
VideoResolution.NUM_4K_8_7
VideoResolution.NUM_4K_8_7_V2
VideoResolution.NUM_4K_9_16_V2
VideoResolution.NUM_5K
VideoResolution.NUM_5K_4_3
VideoResolution.NUM_5_3K
VideoResolution.NUM_5_3K_21_9
VideoResolution.NUM_5_3K_4_3
VideoResolution.NUM_5_3K_4_3_V2
VideoResolution.NUM_5_3K_8_7
VideoResolution.NUM_5_3K_8_7_V2
VideoResolution.NUM_720
VideoResolution.NUM_900
VideoTimelapseRate
VideoTimelapseRate.NUM_0_5_SECONDS
VideoTimelapseRate.NUM_10_SECONDS
VideoTimelapseRate.NUM_1_SECOND
VideoTimelapseRate.NUM_2_MINUTES
VideoTimelapseRate.NUM_2_SECONDS
VideoTimelapseRate.NUM_30_MINUTES
VideoTimelapseRate.NUM_30_SECONDS
VideoTimelapseRate.NUM_3_SECONDS
VideoTimelapseRate.NUM_5_MINUTES
VideoTimelapseRate.NUM_5_SECONDS
VideoTimelapseRate.NUM_60_MINUTES
VideoTimelapseRate.NUM_60_SECONDS
WebcamDigitalLenses
WirelessBand
CameraControlId
DisplayModStatus
DisplayModStatus.NUM_000_DISPLAY_MOD_0_HDMI_0_DISPLAY_MOD_CONNECTED_FALSE
DisplayModStatus.NUM_001_DISPLAY_MOD_0_HDMI_0_DISPLAY_MOD_CONNECTED_TRUE
DisplayModStatus.NUM_010_DISPLAY_MOD_0_HDMI_1_DISPLAY_MOD_CONNECTED_FALSE
DisplayModStatus.NUM_011_DISPLAY_MOD_0_HDMI_1_DISPLAY_MOD_CONNECTED_TRUE
DisplayModStatus.NUM_100_DISPLAY_MOD_1_HDMI_0_DISPLAY_MOD_CONNECTED_FALSE
DisplayModStatus.NUM_101_DISPLAY_MOD_1_HDMI_0_DISPLAY_MOD_CONNECTED_TRUE
DisplayModStatus.NUM_110_DISPLAY_MOD_1_HDMI_1_DISPLAY_MOD_CONNECTED_FALSE
DisplayModStatus.NUM_111_DISPLAY_MOD_1_HDMI_1_DISPLAY_MOD_CONNECTED_TRUE
LastPairingType
LensType
LiveviewExposureSelectMode
MediaModState
MicrophoneAccessory
Ota
PairingState
PrimaryStorage
Rotation
StatusId
StatusId.ACCESS_POINT_SSID
StatusId.ACTIVE_HILIGHTS
StatusId.AP_MODE
StatusId.BATTERY_PRESENT
StatusId.BUSY
StatusId.CAMERA_CONTROL_ID
StatusId.CAPTURE_DELAY_ACTIVE
StatusId.COLD
StatusId.CONNECTED_DEVICES
StatusId.CONNECTED_WIFI_SSID
StatusId.DISPLAY_MOD_STATUS
StatusId.ENCODING
StatusId.FLATMODE
StatusId.FTU
StatusId.GPS_LOCK
StatusId.HINDSIGHT
StatusId.LAST_PAIRING_SUCCESS
StatusId.LAST_PAIRING_TYPE
StatusId.LAST_WIFI_SCAN_SUCCESS
StatusId.LCD_LOCK
StatusId.LENS_TYPE
StatusId.LINUX_CORE
StatusId.LIVEVIEW_EXPOSURE_SELECT_MODE
StatusId.LIVEVIEW_X
StatusId.LIVEVIEW_Y
StatusId.LIVE_BURSTS
StatusId.LOCATE
StatusId.MEDIA_MOD_STATE
StatusId.MICROPHONE_ACCESSORY
StatusId.MINIMUM_STATUS_POLL_PERIOD
StatusId.MOBILE_FRIENDLY
StatusId.NUM_5GHZ_AVAILABLE
StatusId.OTA
StatusId.OTA_CHARGED
StatusId.OVERHEATING
StatusId.PAIRING_STATE
StatusId.PENDING_FW_UPDATE_CANCEL
StatusId.PHOTOS
StatusId.PHOTO_INTERVAL_CAPTURE_COUNT
StatusId.PHOTO_PRESET
StatusId.PRESET
StatusId.PRESET_GROUP
StatusId.PRESET_MODIFIED
StatusId.PREVIEW_STREAM
StatusId.PREVIEW_STREAM_AVAILABLE
StatusId.PRIMARY_STORAGE
StatusId.QUICK_CAPTURE
StatusId.READY
StatusId.REMAINING_LIVE_BURSTS
StatusId.REMAINING_PHOTOS
StatusId.REMAINING_VIDEO_TIME
StatusId.REMOTE_CONNECTED
StatusId.REMOTE_VERSION
StatusId.ROTATION
StatusId.SCHEDULED_CAPTURE
StatusId.SCHEDULED_CAPTURE_PRESET_ID
StatusId.SD_CARD_CAPACITY
StatusId.SD_CARD_ERRORS
StatusId.SD_CARD_REMAINING
StatusId.SD_CARD_WRITE_SPEED_ERROR
StatusId.TIMELAPSE_INTERVAL_COUNTDOWN
StatusId.TIMELAPSE_PRESET
StatusId.TIME_SINCE_LAST_HILIGHT
StatusId.TIME_WARP_SPEED
StatusId.TURBO_TRANSFER
StatusId.USB_CONNECTED
StatusId.USB_CONTROLLED
StatusId.VIDEOS
StatusId.VIDEO_ENCODING_DURATION
StatusId.VIDEO_PRESET
StatusId.WIFI_BARS
StatusId.WIFI_PROVISIONING_STATE
StatusId.WIFI_SCAN_STATE
StatusId.WIRELESS_BAND
StatusId.WIRELESS_CONNECTIONS_ENABLED
StatusId.ZOOM_AVAILABLE
StatusId.ZOOM_LEVEL
StatusId.ZOOM_WHILE_ENCODING
TimeWarpSpeed
TimeWarpSpeed.AUTO
TimeWarpSpeed.NUM_10X
TimeWarpSpeed.NUM_150X
TimeWarpSpeed.NUM_15X
TimeWarpSpeed.NUM_1800X
TimeWarpSpeed.NUM_1X_REALTIME_
TimeWarpSpeed.NUM_1_2X_SLOW_MOTION_
TimeWarpSpeed.NUM_2X
TimeWarpSpeed.NUM_300X
TimeWarpSpeed.NUM_30X
TimeWarpSpeed.NUM_5X
TimeWarpSpeed.NUM_60X
TimeWarpSpeed.NUM_900X
UsbControlled
WifiProvisioningState
WifiScanState
WirelessBand
- Exceptions
- Common Interface
- BLE Interface
- WiFi Interface
- Troubleshooting
- Contributing
- Credits
- Changelog
- 0.20.0 (May-12-2025)
- 0.19.8 (April-30-2025)
- 0.19.7 (April-28-2025)
- 0.19.6 (April-8-2025)
- 0.19.5 (March-27-2025)
- 0.19.4 (March-21-2025)
- 0.19.3 (March-20-2025)
- 0.19.2 (March-19-2025)
- 0.19.1 (March-18-2025)
- 0.19.0 (February-20-2025)
- 0.18.0 (January-7-2025)
- 0.17.1 (September-13-2024)
- 0.17.0 (September-9-2024)
- 0.16.2 (July-18-2024)
- 0.16.1 (April-23-2024)
- 0.16.0 (April-9-2024)
- 0.15.1 (December-6-2023)
- 0.15.0 (December-6-2023)
- 0.14.1 (September-21-2023)
- 0.14.0 (September-13-2023)
- 0.13.0 (February-24-2023)
- 0.12.0 (December-16-2023)
- 0.11.2 (November-9-2022)
- 0.11.1 (October-18-2022)
- 0.11.0 (September-14-2022)
- 0.10.0 (July-14-2022)
- 0.9.2 (June-16-2022)
- 0.9.1 (May-27-2022)
- 0.9.0 (February-7-2022)
- 0.8.0 (February-3-2022)
- 0.7.2 (January-3-2022)
- 0.7.1 (December-16-2021)
- 0.7.0 (October-27-2021)
- 0.6.3 (October-7-2021)
- 0.6.2 (September-28-2021)
- 0.6.1 (September-20-2021)
- 0.6.0 (September-2-2021)
- 0.5.8 (August-10-2021)
- 0.5.7 (June-7-2021)
- 0.5.6 (May-26-2021)
- 0.5.5 (May-26-2021)
- 0.5.4 (May-6-2021)
- 0.5.3 (April-15-2021)
- 0.5.2 (April-2-2021)
- 0.5.1 (April-1-2021)
- 0.5.0 (March-30-2021)
- 0.4.6 (March-29-2021)
- 0.4.5 (March-29-2021)
- 0.4.4 (March-27-2021)
- 0.4.3 (March-26-2021)
- 0.4.2 (March-25-2021)
- 0.4.1 (March-25-2021)
- 0.4.0 (March-25-2021)
- 0.3.3 (March-22-2021)
- 0.3.2 (March-15-2021)
- 0.3.1 (March-12-2021)
- 0.3.0 (March-11-2021)
- 0.2.0 (March-10-2021)
- 0.1.x (March-10-2021)
- Future Work